📗 Django
Django MTV with RUD
==본문 만들기
다시 생각해 보면
이렇게 새로운 제목과 내용을 작성하고, 저장을 누르면
이 화면을 보여주진 않는다.
🤔 create.html를 인배딩 과정을 어떻게 없앨 수 있을까?
redirect 사용하기
- redirect는 지정한 URL로 되돌리는 것
- 우리가 웹 사이트를 이용하면서 많이 봐왔던 동작 방식이다.
↓ 해보자
더보기

변경 전


변경 후


articles/views.py

⬇️


from django.shortcuts import render, redirect
def create(request):
title = request.POST.get("title")
content = request.POST.get("content")
article = Article(title=title, content=content)
article.save()
return redirect('articles')
- import에 redirect를 추가해준다.
- return redirect('name')로 수정해주고 변경해주고자 하는 url name을 써준다.
서버 실행하기


이제 creat.html은 필요 없어졌으니 삭-제
상세페이지 조회하기
생성된 게시글을 읽는 url를 만들어주자
구현하기
더보기


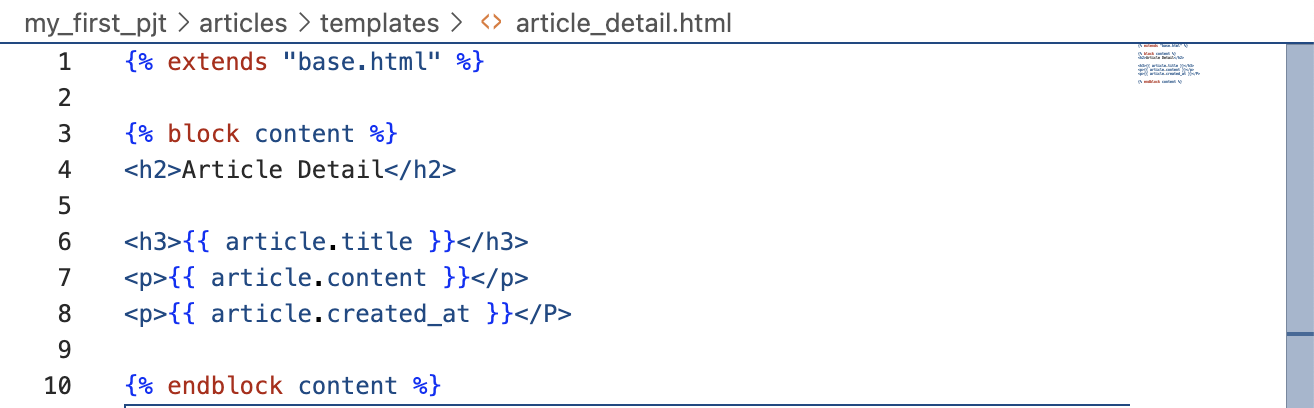


1️⃣ urls.py에 추가해 주기

path('<int:pk>/', views.article_detail, name="article_detail"),
2️⃣ views.py에 article_detail 함수 추가하기

def article_detail(request, pk):
article = Article.objects.get(pk=pk)
context = {"article": article}
return render(request, "article_detail.html", context)
- Article 모델을 통해서 pk에 해당되는 내용을 보여주려고 작성한 코드이다.
3️⃣ article_detail templates 작성하기
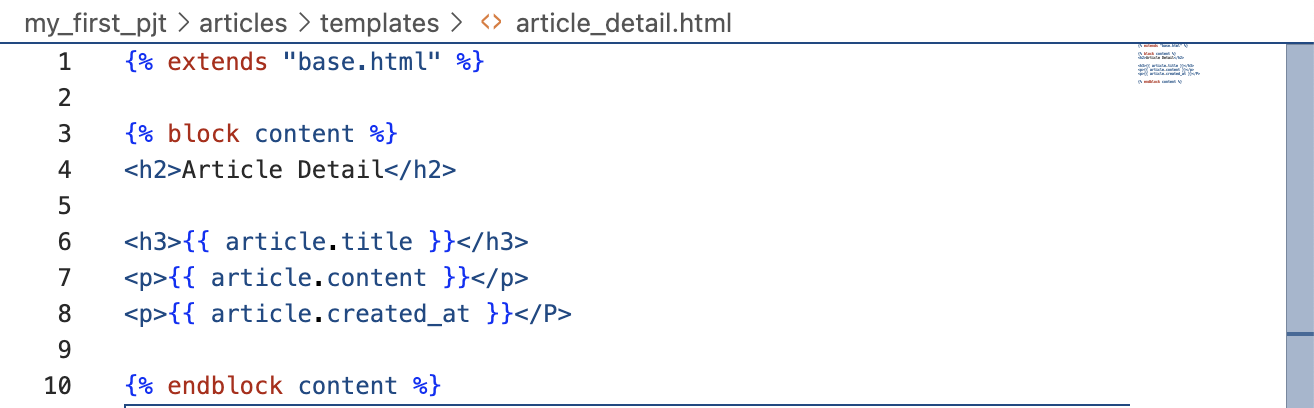
{% extends "base.html" %}
{% block content %}
<h2>Article Detail</h2>
<h3>{{ article.title }}</h3>
<p>{{ article.content }}</p>
<p>{{ article.created_at }}</P>
{% endblock content %}
4️⃣ server 가서 url에 숫자 입력하기


되네!
추가 작업
게시글 작성 후 상세 페이지로 리다이렉트 시키는 걸 구현
더보기

변경 전

views.py of articles app
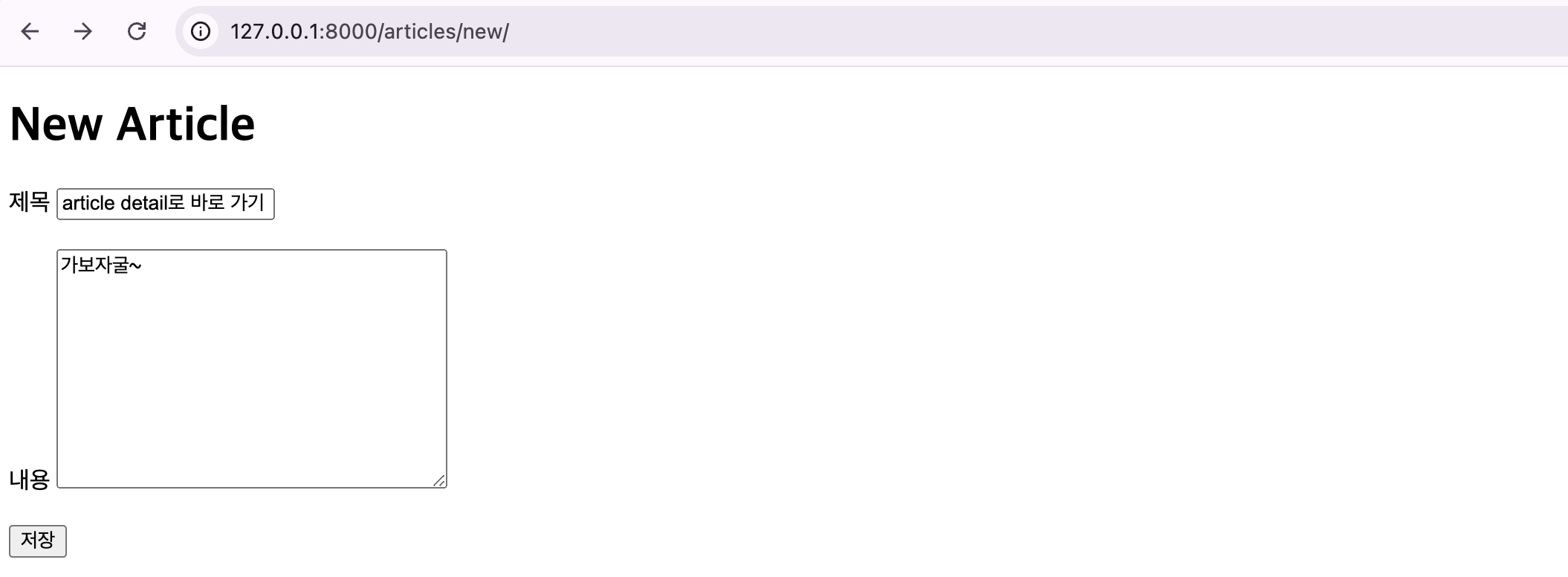


⬇️

def create(request):
title = request.POST.get("title")
content = request.POST.get("content")
article = Article.objects.create(title=title, content=content)
article.save()
return redirect('article_detail', article.pk)
변경 사항
return redirect('article_detail', article.pk)
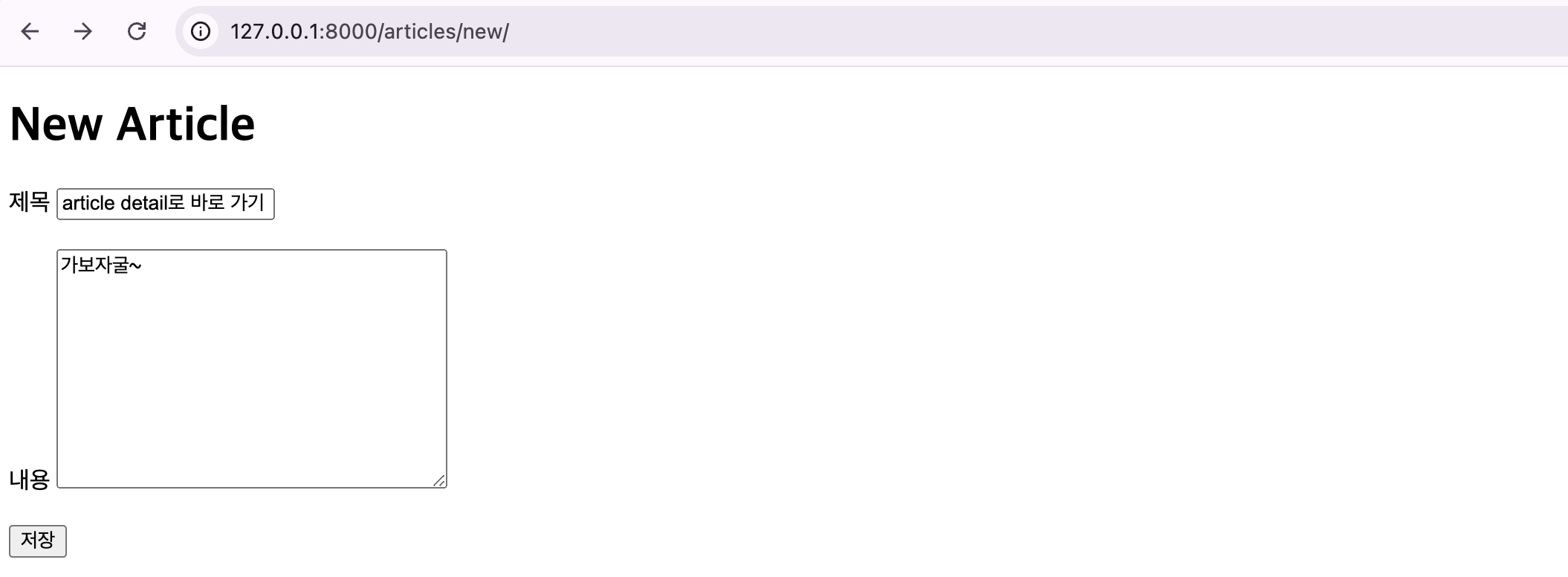

되네!
편의성 업데이트
⬇️ Article 화면에 글 작성 링크 만들기
더보기

변경 전



⬇️

{% extends "base.html" %}
{% block content %}
<h2>Articles</h2>
<a href='{% url 'new_article' %}'>Writing a new article</a>
{% for article in articles %}
<p>[{{ article.id }}] {{ article.title }} : {{ article.content }}</p>
<hr>
{% endfor %}
<a href="{% url 'new_article' %}">Make a new article</a><br><br>
{% endblock content %}
변경 사항
<a href='{% url 'new' %}'>Writing a new article</a>

생겼넹!
⬇️ new_article 화면에도 article 목록으로 가는 링크 만들기
더보기

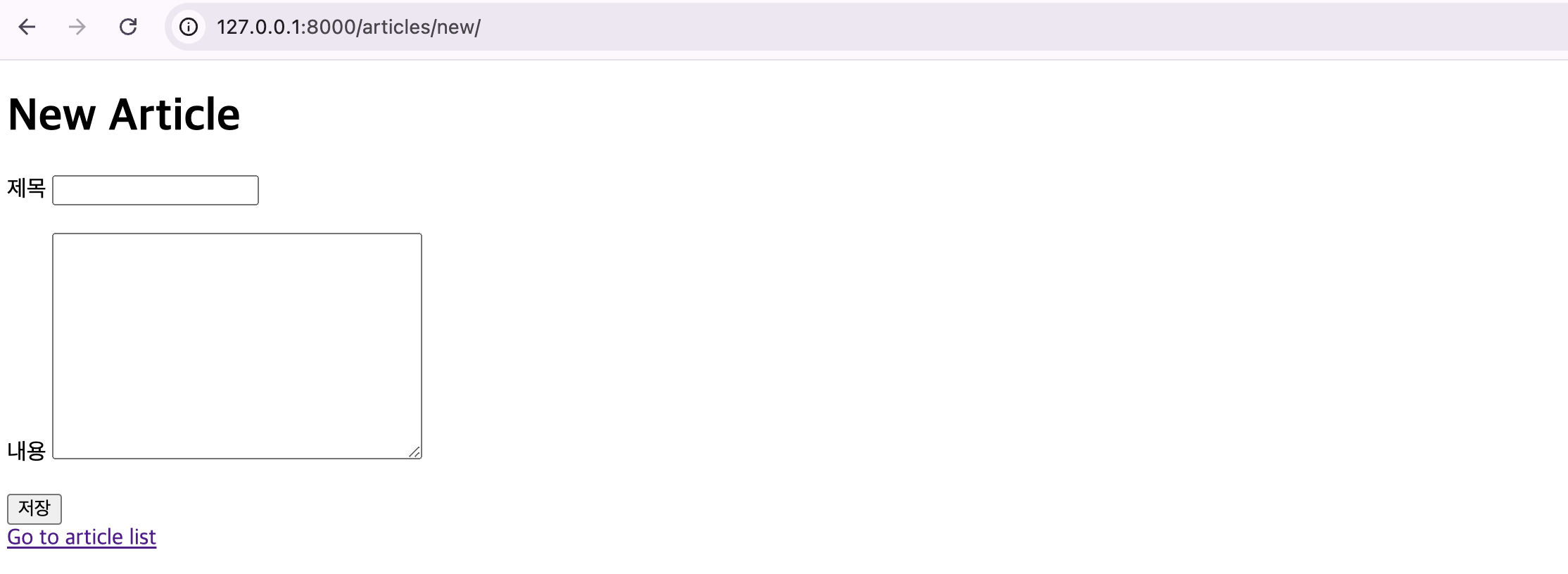

{% extends "base.html" %}
{% block content %}
<h1>New Article</h1>
<form action="{% url 'create' %}" method="POST">
{% csrf_token%}
<label for="title">제목</label>
<input type="text" name="title" id="title"><br><br>
<label for="content">내용</label>
<textarea name="content" id="content" cols="30" rows="10"></textarea><br><br>
<button type="submit">저장</button>
</form>
<a href='{% url 'articles' %}'>Go to article list</a>
{% endblock content %}
변경 사항
<a href='{% url 'articles' %}'>Go to article list</a>
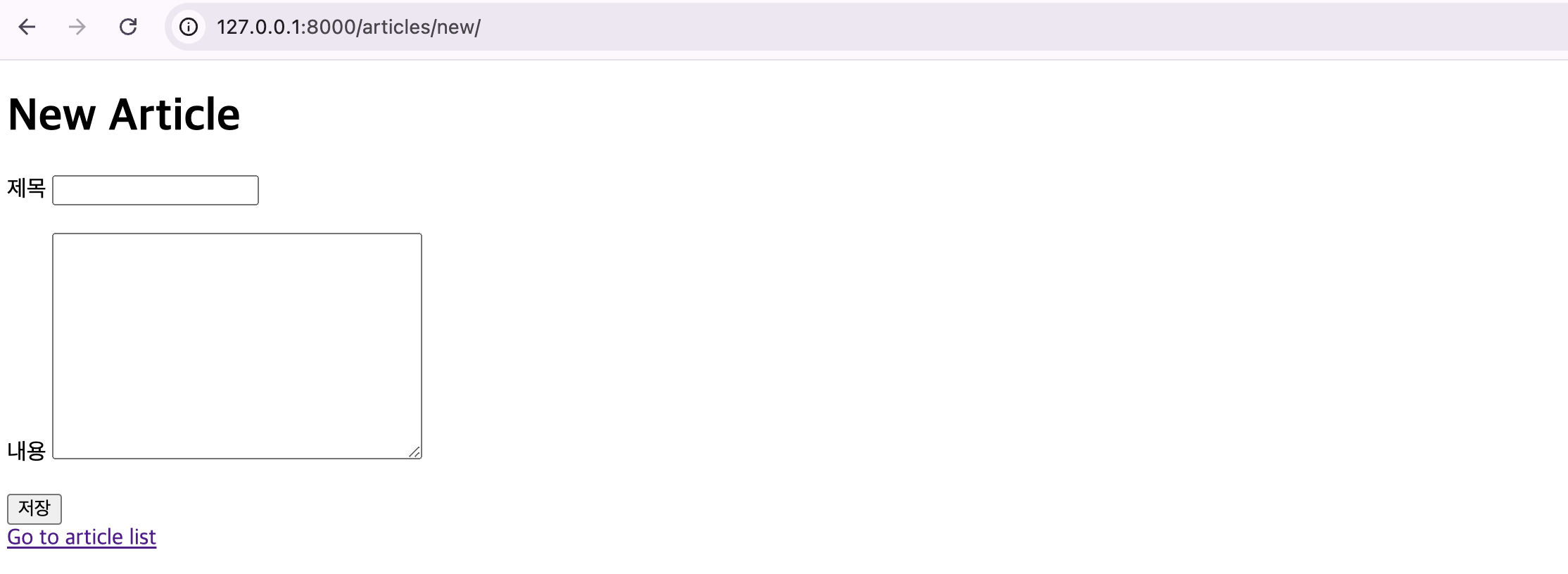
생겼넹!
⬇️ articles 목록을 게시판처럼 만들기
더보기

변경 전
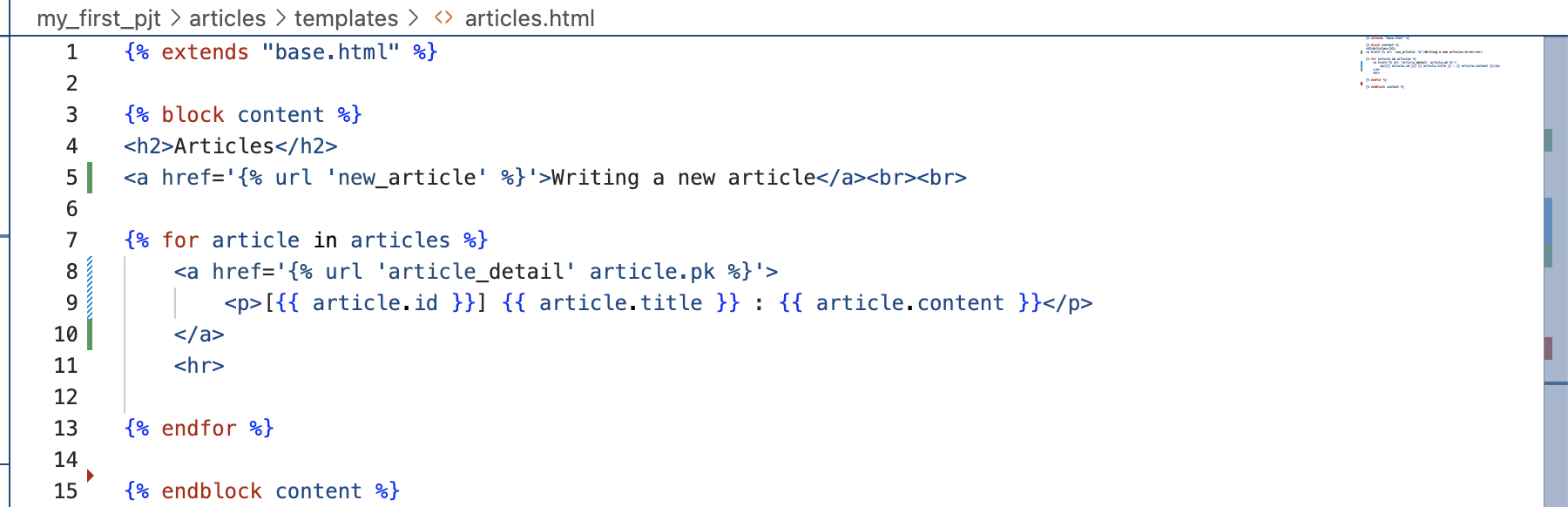
변경 후



⬇️
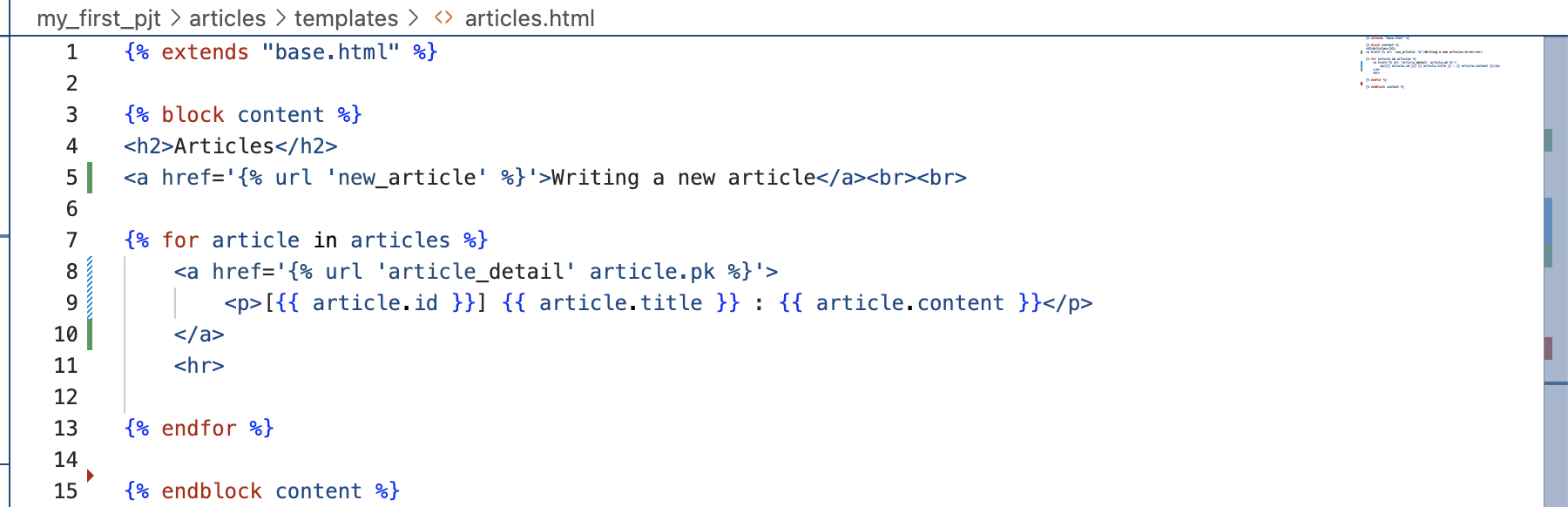
{% extends "base.html" %}
{% block content %}
<h2>Articles</h2>
<a href='{% url 'new_article' %}'>Writing a new article</a><br><br>
{% for article in articles %}
<a href='{% url 'article_detail' article.pk %}'>
<p>[{{ article.id }}] {{ article.title }} : {{ article.content }}</p>
</a>
<hr>
{% endfor %}
{% endblock content %}
변경 사항
# 추가
<a href='{% url 'article_detail' article.pk %}'>
# 삭제
<a href="{% url 'new_article' %}">Make a new article</a><br><br>


바꼈넹!
⬇️ 글 작성 링크에 버튼 만들기
더보기
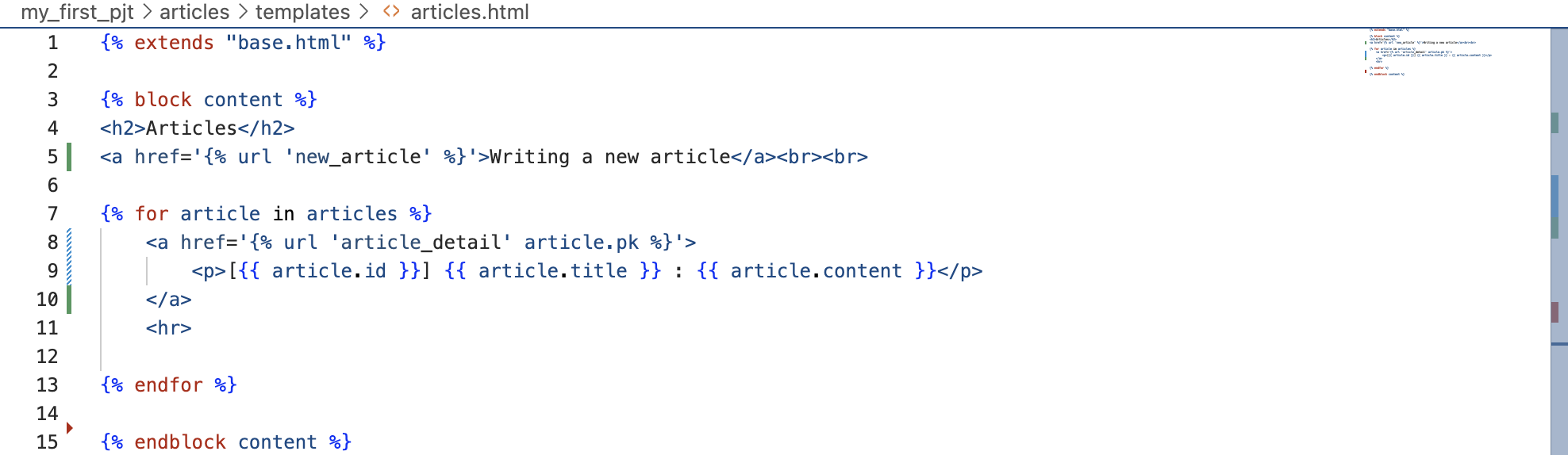
변경 전
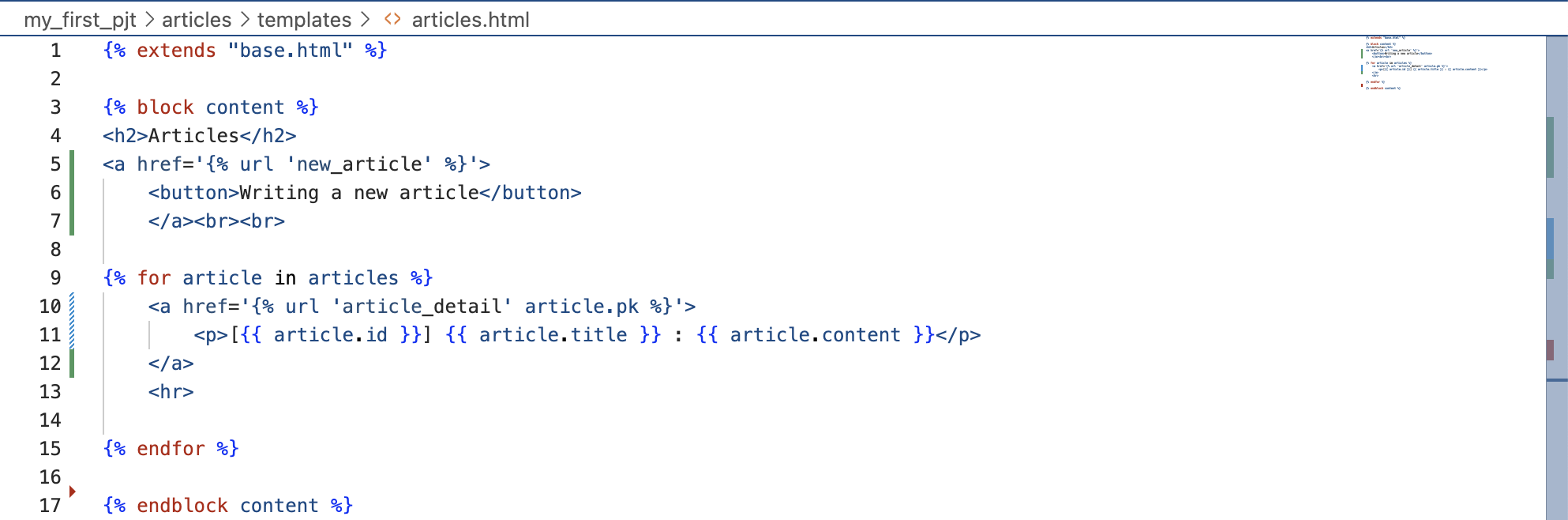
변경 후

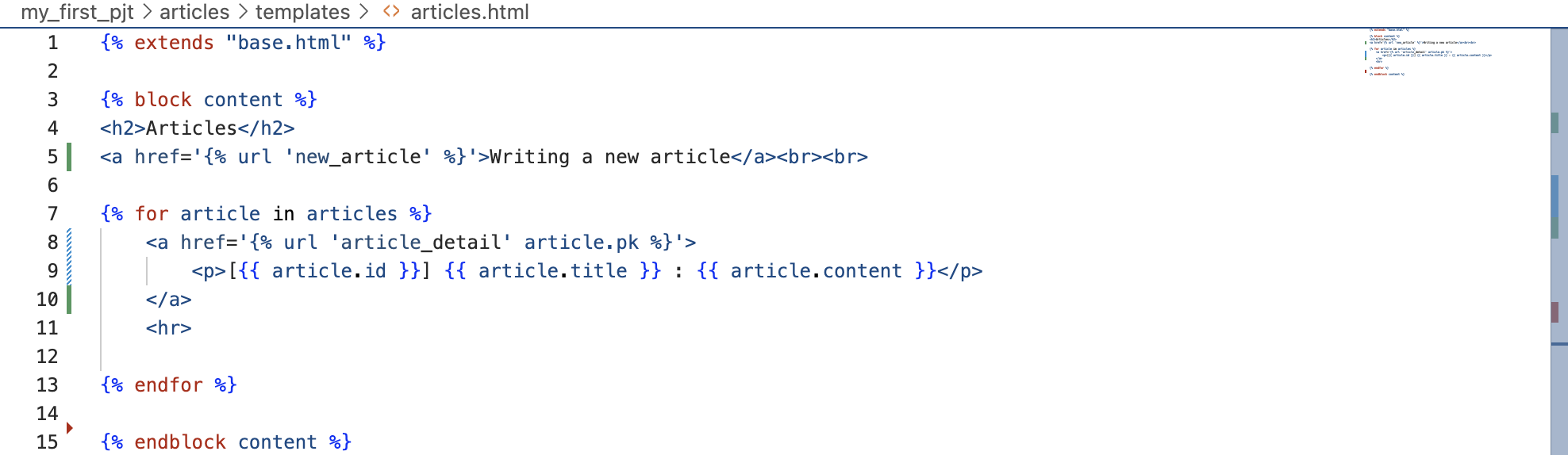
⬇️
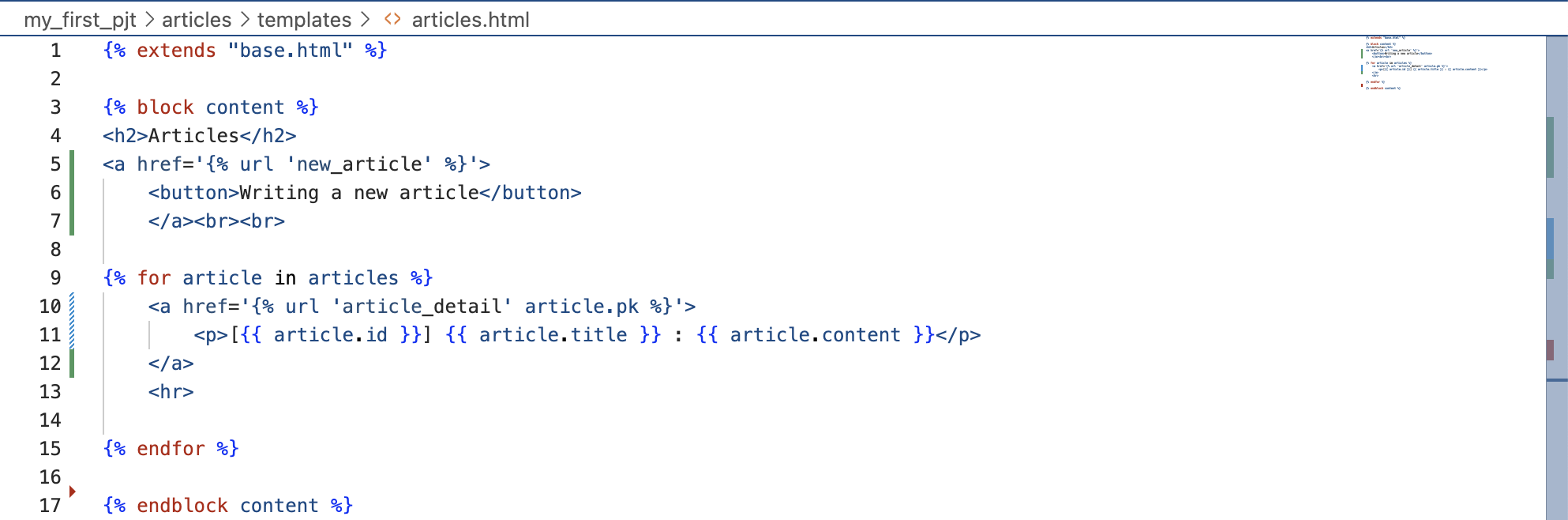
변경 사항
<a href='{% url 'new_article' %}'>
<button>Writing a new article</button>
</a><br><br>

생겼넹!
글 삭제하기
- 구현
- 글 삭제 로직을 진행하는 url 만들기
- 글 삭제하는 view 만들기
- 삭제하고자 하는 글 가져오기
- 글 삭제
- 삭제한 다음 이동할 곳으로 redirect
- 글 삭제 버튼 만들어주기
↓ 해보자
더보기


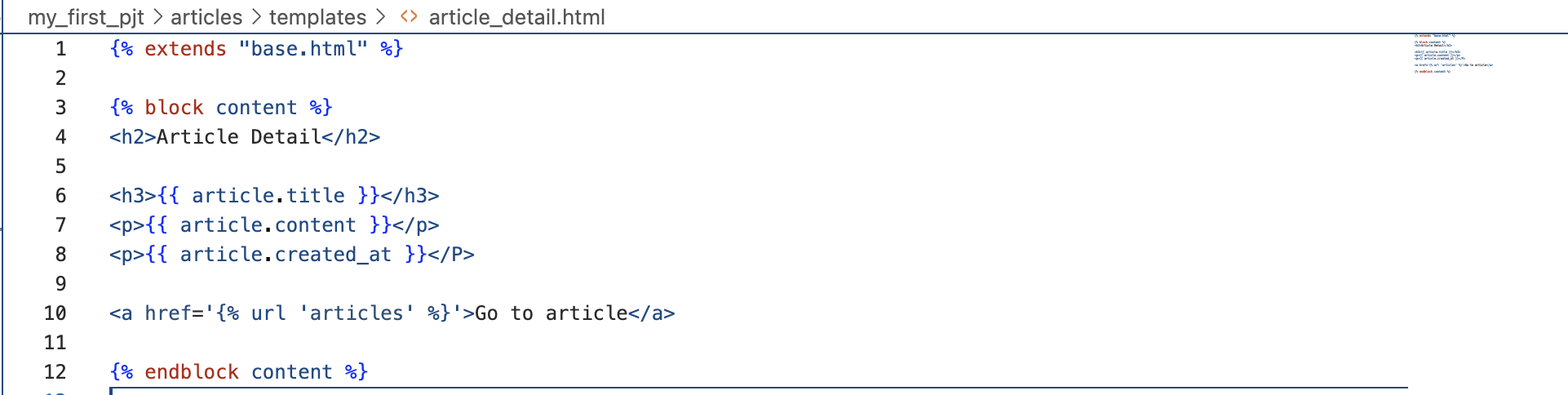
변경 전

변경 후

1️⃣ urls.py에 삭제 url 만들기

path('<int:pk>/delete/', views.delete, name="delete"),
2️⃣ views.py에 함수 추가하기

def delete(request, pk):
if request.method == "POST":
article = Article.objects.get(pk=pk)
article.delete()
return redirect("articles")
return redirect('article_detail', pk)
- Method가 POST일 경우에만 삭제가 되도록 했다.
- Method가 GET일 경우에는 실행되지 않는다.
3️⃣ article_detail.html에서 삭제 버튼 만들어 주기
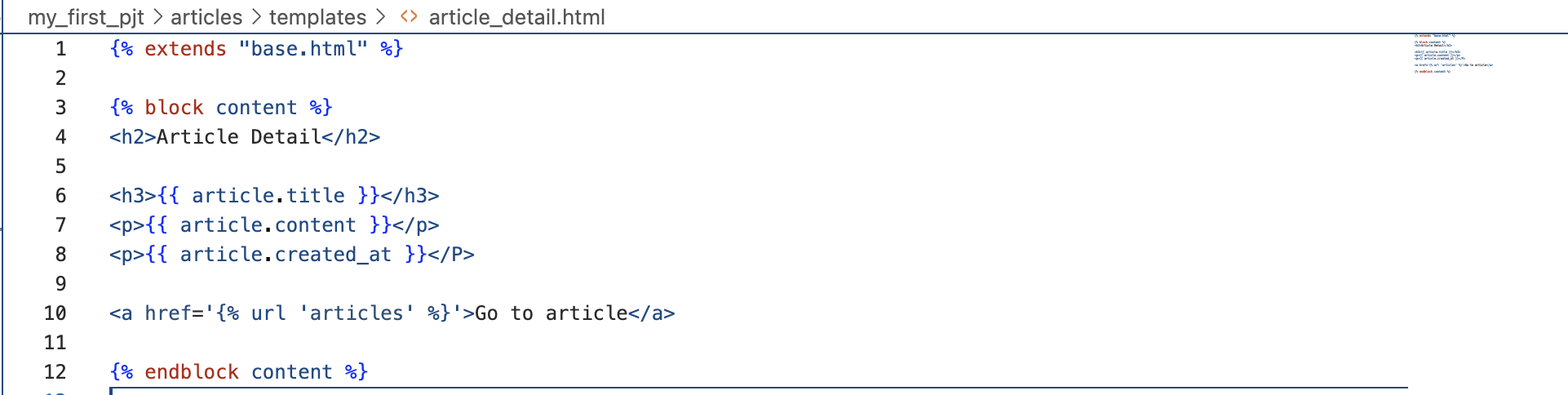
⬇️

{% extends "base.html" %}
{% block content %}
<h2>Article Detail</h2>
<h3>{{ article.title }}</h3>
<p>{{ article.content }}</p>
<p>{{ article.created_at }}</P>
<a href='{% url 'articles' %}'>Go to article</a><br><br>
<form action="{% url 'delete' article.pk %}" method="POST">
{% csrf_token %}
<input type="submit" value="delete">
</form>
{% endblock content %}
변경 사항
<form action="{% url 'delete' article.pk %}" method="POST">
{% csrf_token %}
<input type="submit" value="delete">
</form>

생겼넹!
글 수정하기
- 글 수정하는 url 만들기
-
- 수정할 글을 보여주는 url
- 글 수정을 처리할 url
- 글 수정하는 로직을 수행하는 view 만들기
- 수정할 글을 보여주는 view
- 글 수정을 처리하는 view
- 글 수정을 수행하는 template 만들기
- 글 수정 버튼 만들기
-
↓ 해보자
더보기

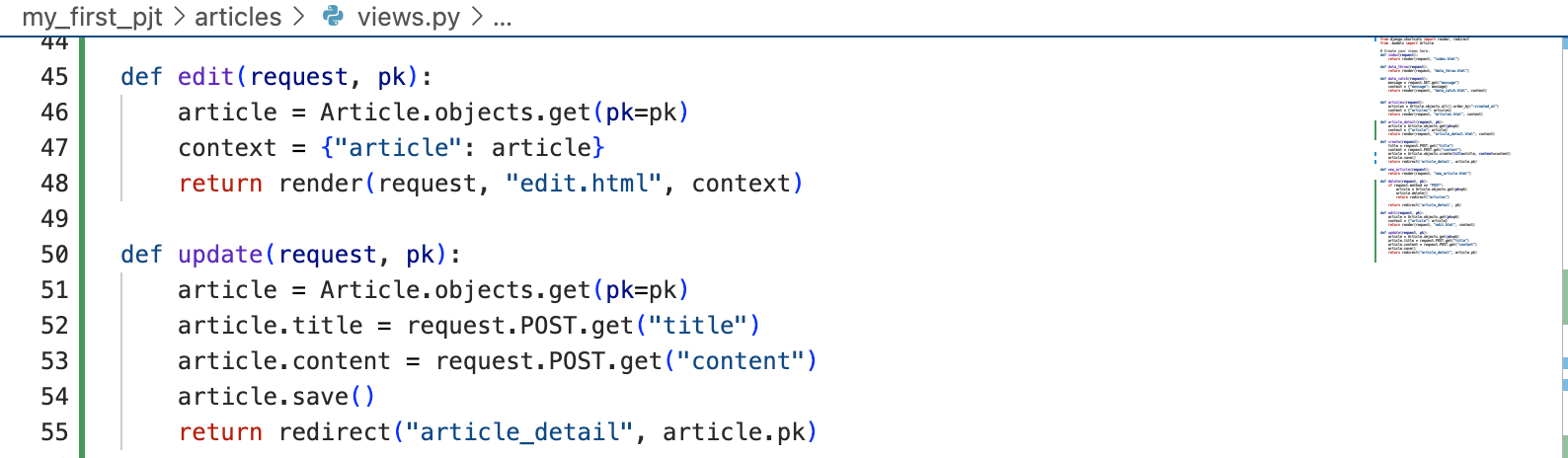





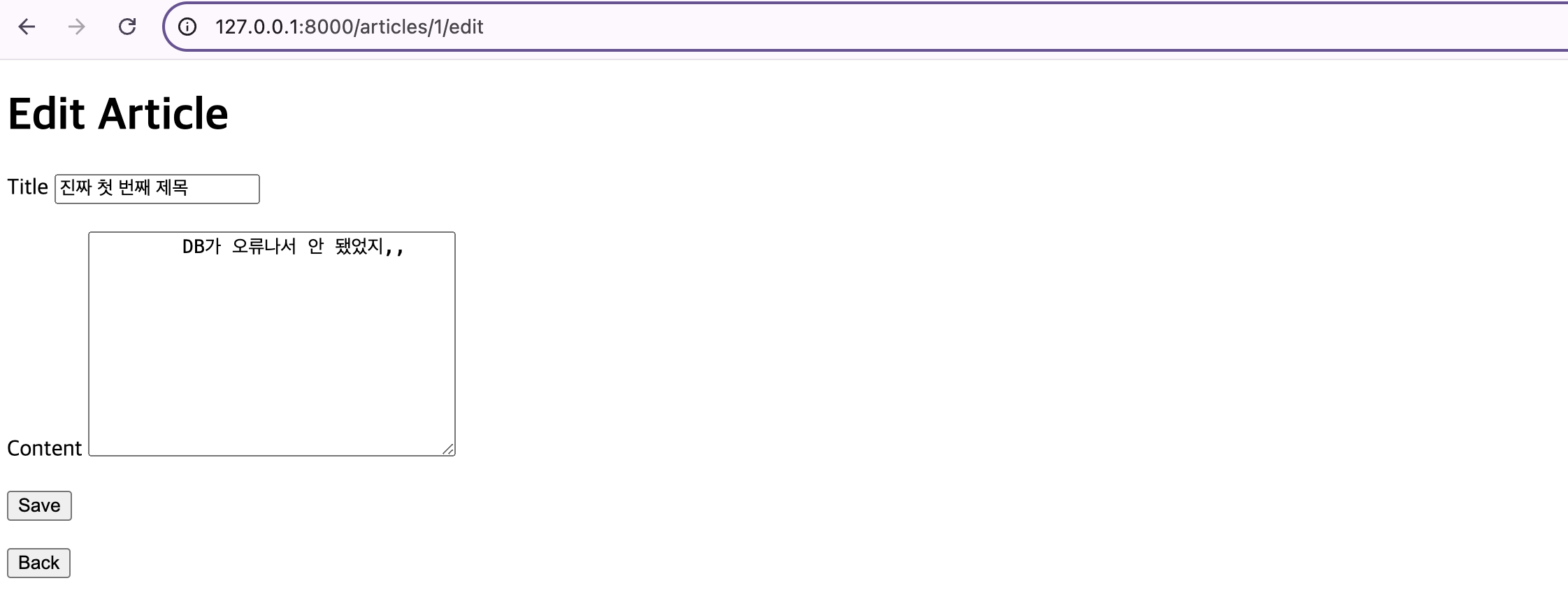
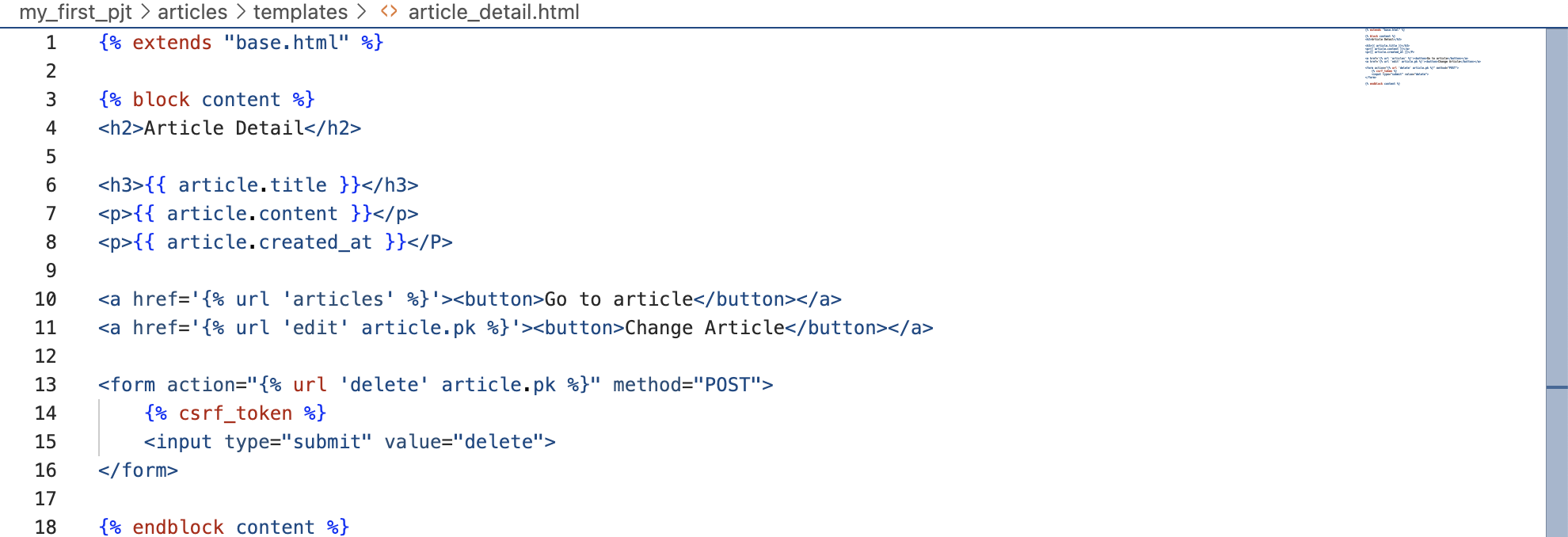

1️⃣ urls.py에 수정할 글 보여주는 url 및 글 수정 처리할 url 추가하기

path('<int:pk>/edit', views.edit, name="edit"),
path('<int:pk>/update', views.update, name="update"),
- edit : 수정할 글 보여주는 url
- update : 글 수정을 처리할 url
2️⃣ 각각의 views.py 함수 만들어 주기
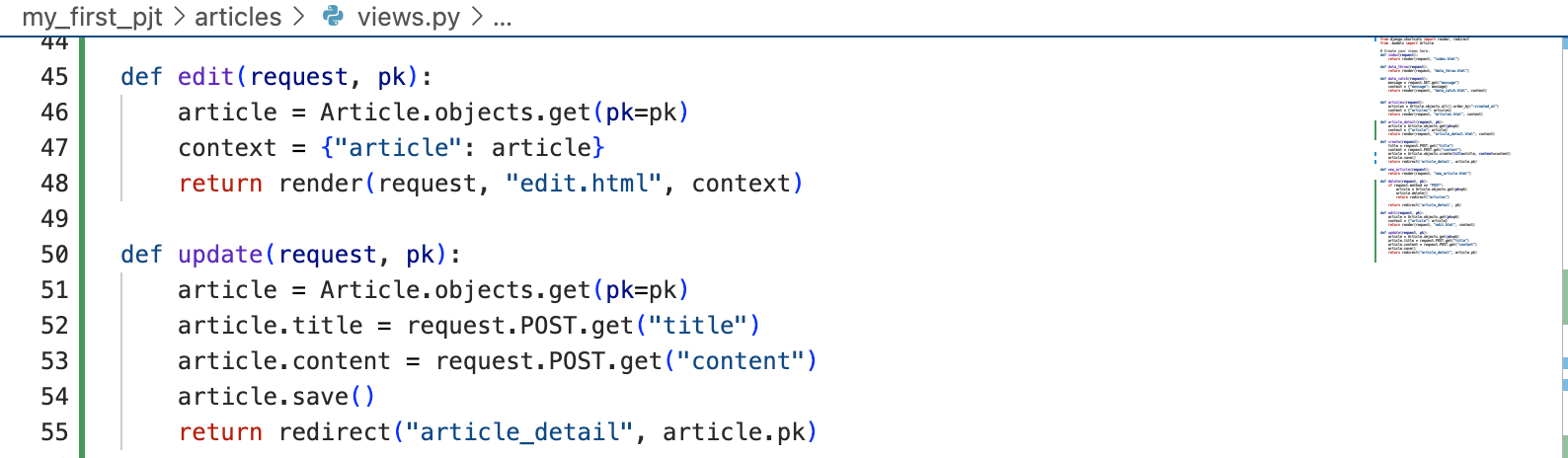
def edit(request, pk):
article = Article.objects.get(pk=pk)
context = {"article": article}
return render(request, "edit.html", context)
def update(request, pk):
article = Article.objects.get(pk=pk)
article.title = request.POST.get("title")
article.content = request.POST.get("content")
article.save()
return redirect("article_detail", article.pk)
3️⃣ edit.html랑 update.html 만들어주기
edit.html

{% extends "base.html" %}
{% block content %}
<h1>Edit Article</h1>
<form action="{% url 'update' article.pk %}" method="POST">
{% csrf_token %}
<label for="title">Title</label>
<input type="text" name="title" id="title" value="{{ article.title }}"><br><br>
<label for="content">Content</label>
<textarea name="content" id="content" cols="30" rows="10">
{{ article.content }}</textarea><br><br>
<button type="submit">Save</button><br><br>
</form>
<a href="{% url "article_detail" article.pk %}">Back</a>
{% endblock content %}
update.html

{% extends "base.html" %}
{% block content %}
<a href="{% url 'edit' article.pk %}"><button>Change Article</button></a>
{% endblock content %}


되네!
각각 버튼 만들어주기
edit.html

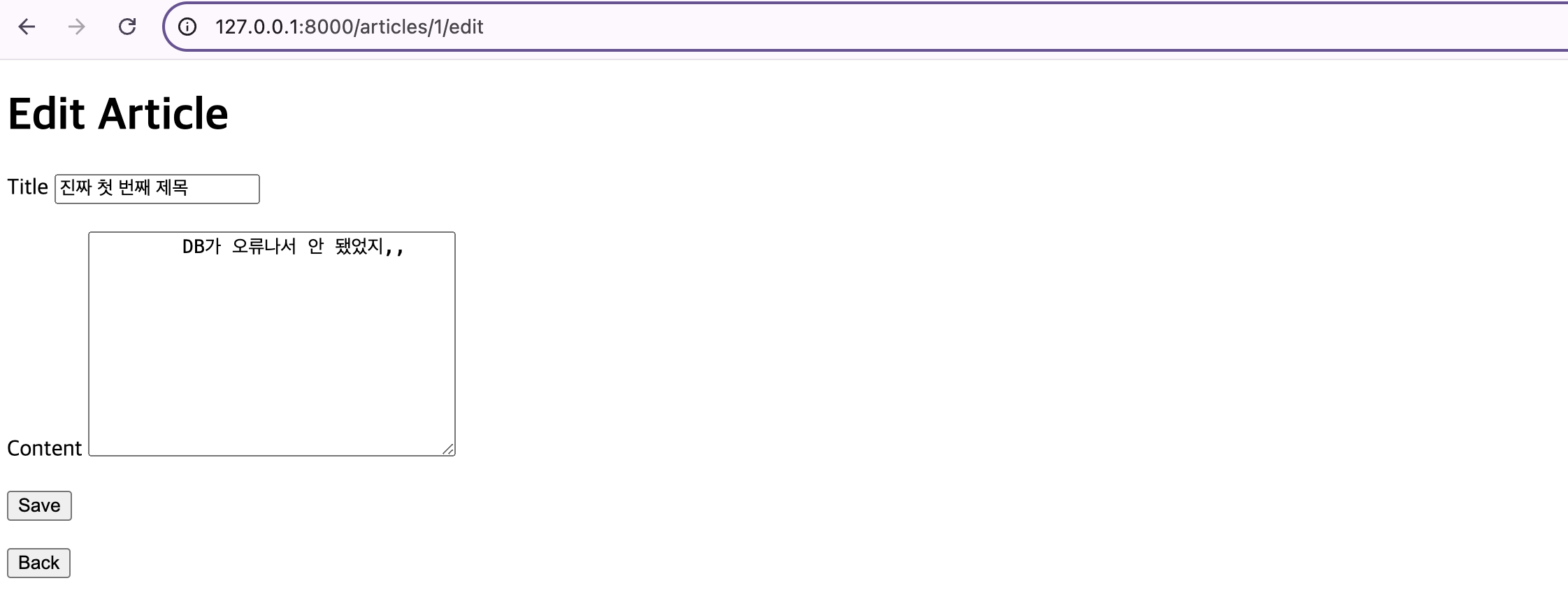
article_detail.html
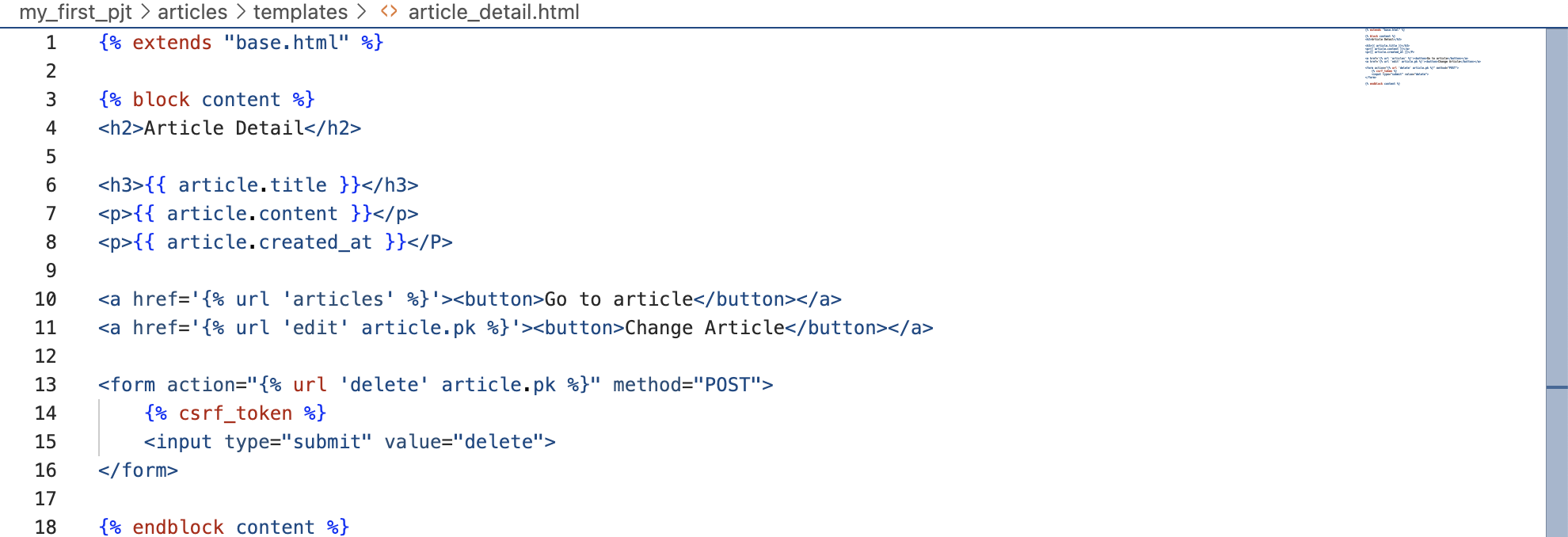
{% extends "base.html" %}
{% block content %}
<h2>Article Detail</h2>
<h3>{{ article.title }}</h3>
<p>{{ article.content }}</p>
<p>{{ article.created_at }}</P>
<a href='{% url 'articles' %}'><button>Go to article</button></a>
<a href='{% url 'edit' article.pk %}'><button>Change Article</button></a>
<form action="{% url 'delete' article.pk %}" method="POST">
{% csrf_token %}
<input type="submit" value="delete">
</form>
{% endblock content %}

오늘의 코드들이 반영된 Server 모습
더보기


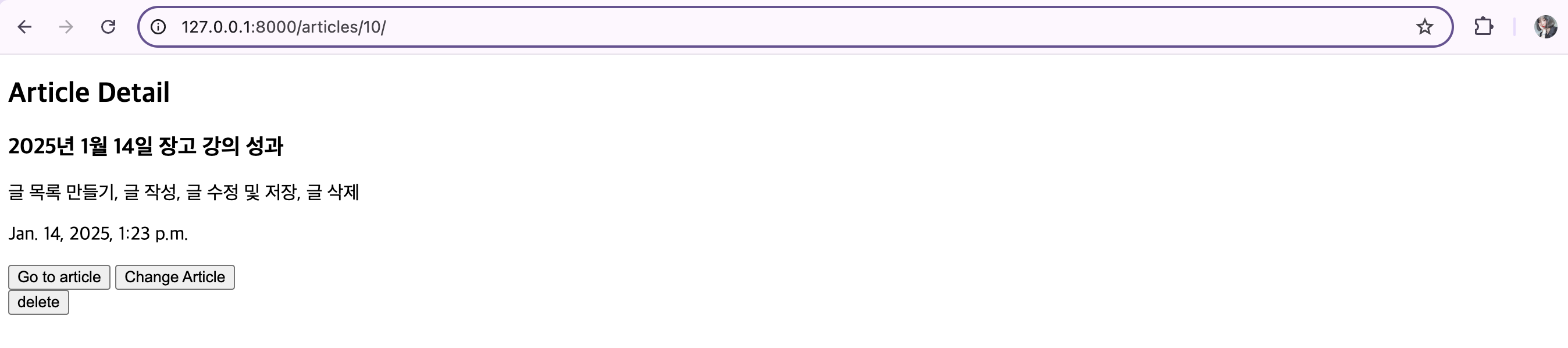


"화요일" 추가함
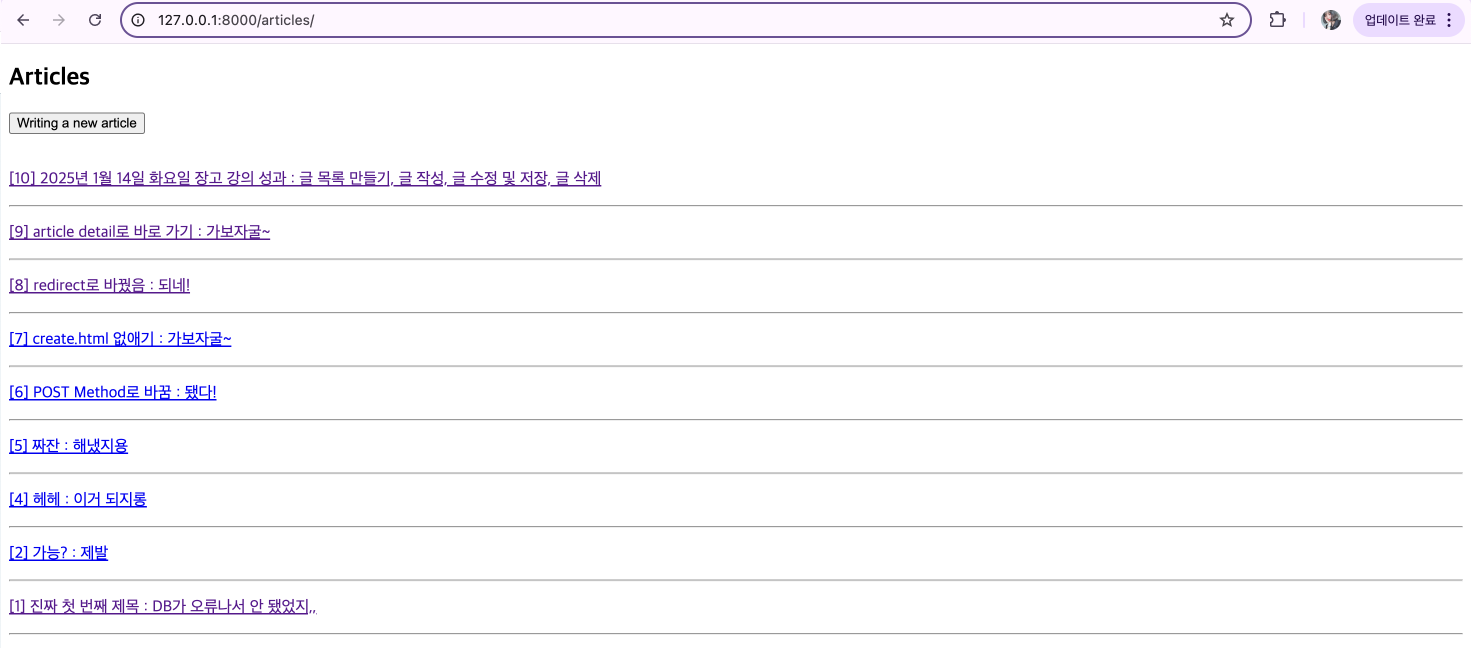


목록 리스트

글 작성

글 저장
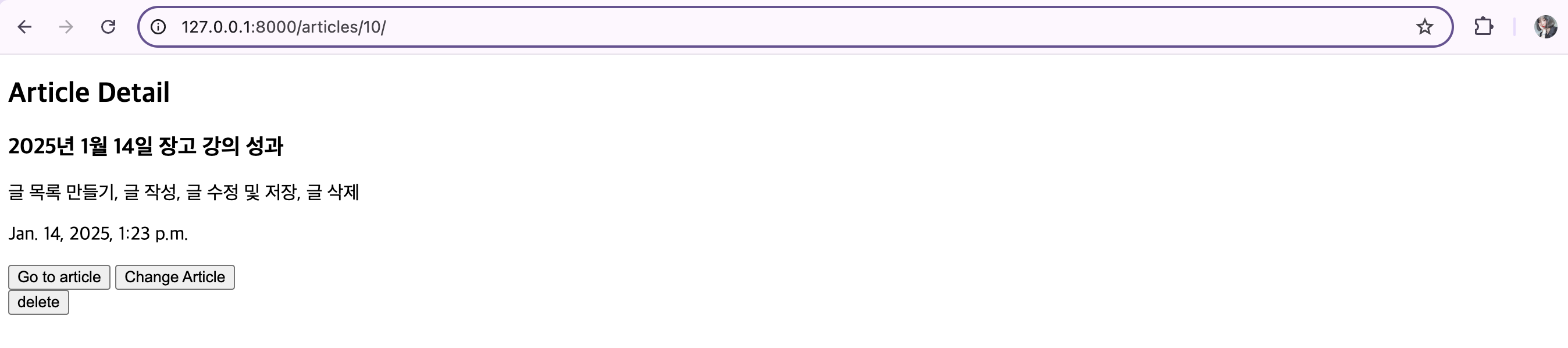
글 수정

글 수정한 거 반영

글 추가한 것과 수정한 게 목록에 잘 반영됐는지 확인
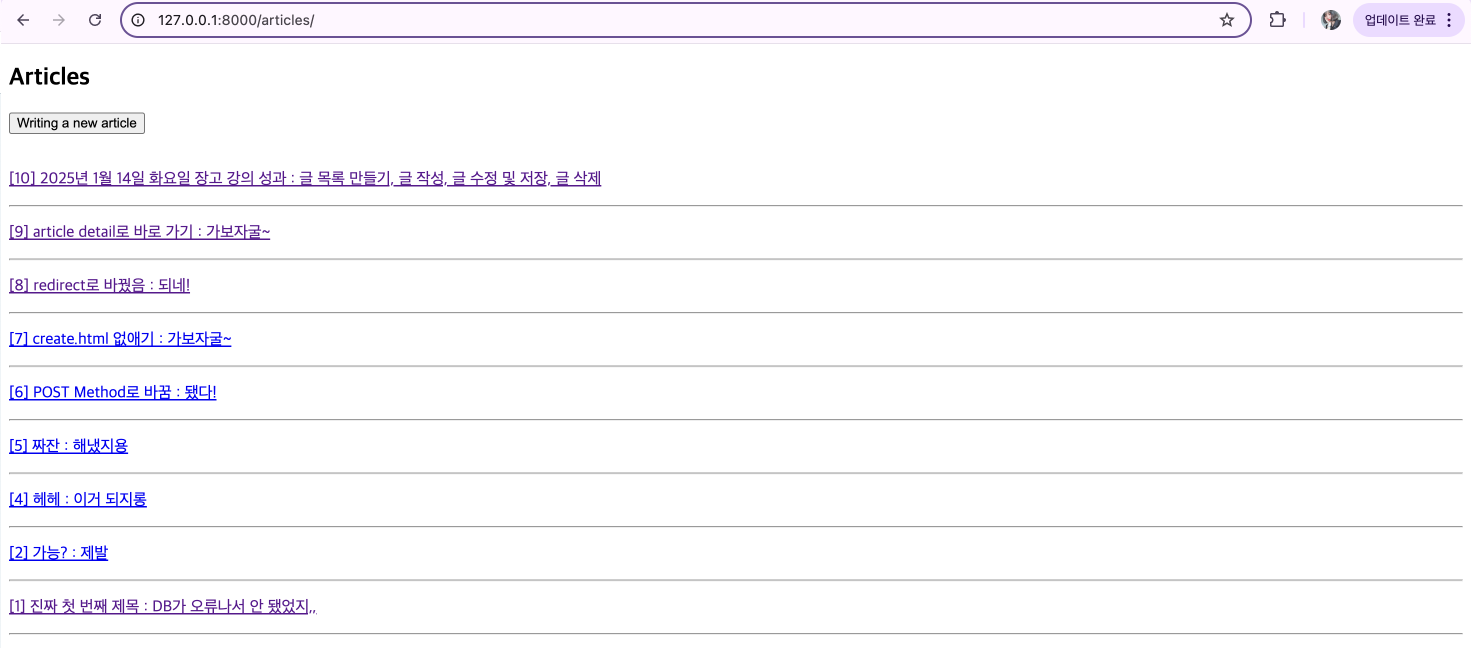
글 삭제하기


'공부 > Django 공부' 카테고리의 다른 글
[Django] Namespace, Templates 구조 (0) | 2025.01.15 |
---|---|
[Django] Django Form Class, Model Form Class (0) | 2025.01.15 |
[Django] Django MTV 사용하기 (CR), POST Method (0) | 2025.01.14 |
[Django] Django ORM, CRUD with Shell (0) | 2025.01.14 |
[Django] Django Model, 마이그레이션 (0) | 2025.01.13 |