📗 Django
Django MTV with CR
== 목록 만들기
↓ 새로운 URL 만들기
더보기
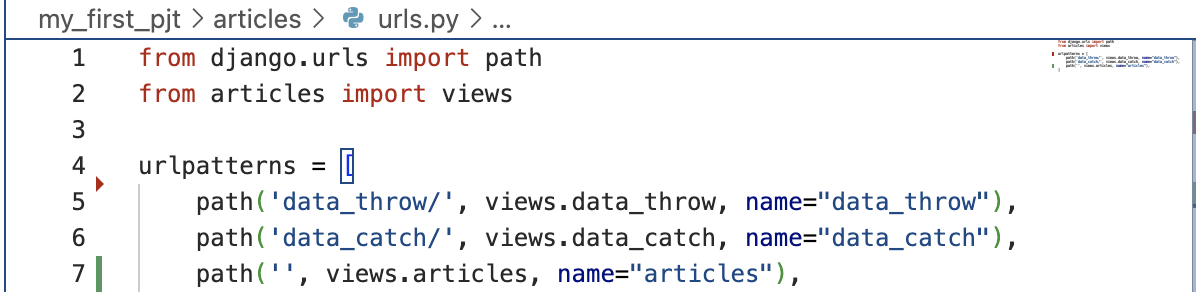

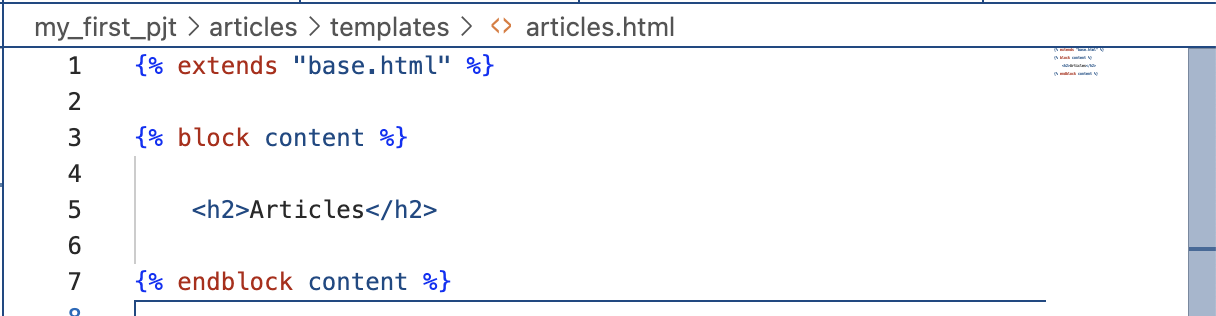
⛳️ /articles/ 로 들어오면 articles.html 템플릿이 랜더링 되어 보이도록 만들기
1️⃣ urls.py에 추가하기
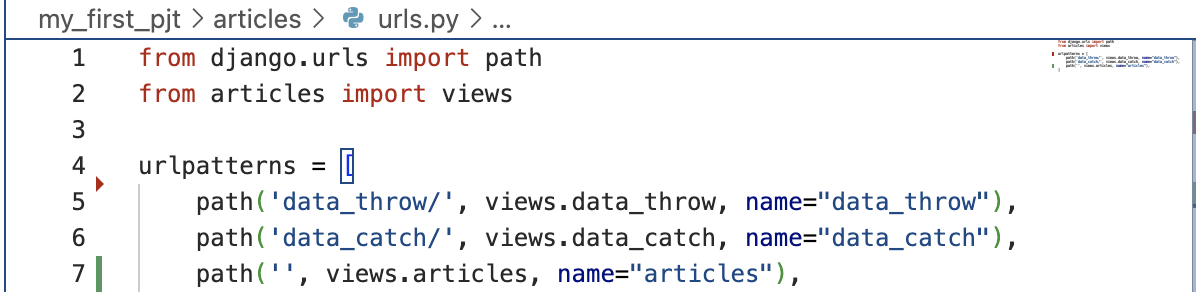
path('', views.articles, name="articles"),
2️⃣ views.py에 함수 추가하기

def articles(request):
return render(request, "articles.html")
3️⃣ articles.html 작성하기
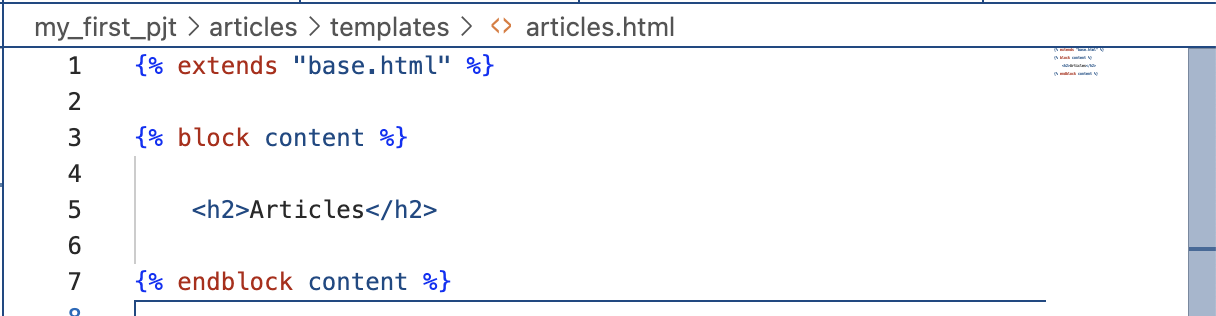
{% extends "base.html" %}
{% block content %}
<h2>Articles</h2>
{% endblock content %}
조회하기
데이터베이스에서 모든 아티클을 조회해서/articles/에서 볼 수 있도록 설정하기
순서
1. view에서 model에 접근해 모든 아티클 가져오기
더보기
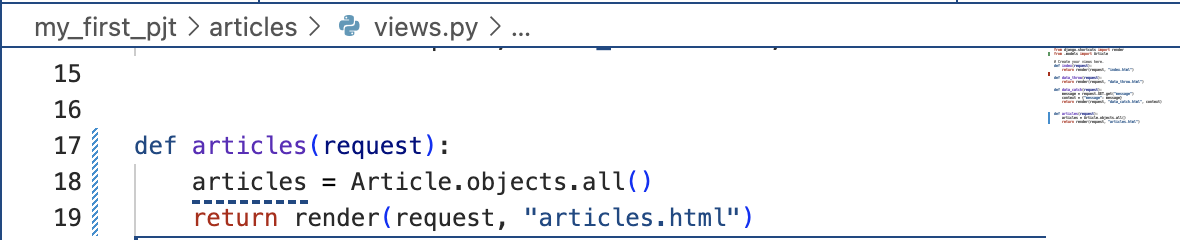
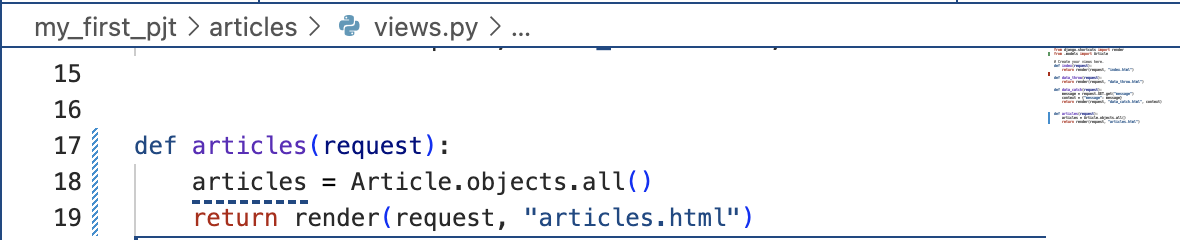
def articles(request):
articles = Article.objects.all()
return render(request, "articles.html")
2. view에서 가져온 아티클을 template으로 넘기기
더보기


def articles(request):
articles = Article.objects.all()
content = {"articles": articles}
return render(request, "articles.html", content)
3. template에서 넘어온 context 보여주기
더보기


{% extends "base.html" %}
{% block content %}
<h2>Articles</h2>
{% for article in articles %}
<p>글 번호 : {{ article.id }}</p>
<p>글 제목 : {{ article.title }}</p>
<p>글 내용 : {{ article.content }}</p>
{% endfor %}
{% endblock content %}
4. view에서 템플릿을 랜더링 해서 리턴
새로운 글 작성 (Form)
/articles/new/로 들어오면 아래의 화면이 보이도록 작성해 보세요!
→ 작성된 form을 보내는 곳은 /articles/create/입니다.
↓ 해보기
더보기

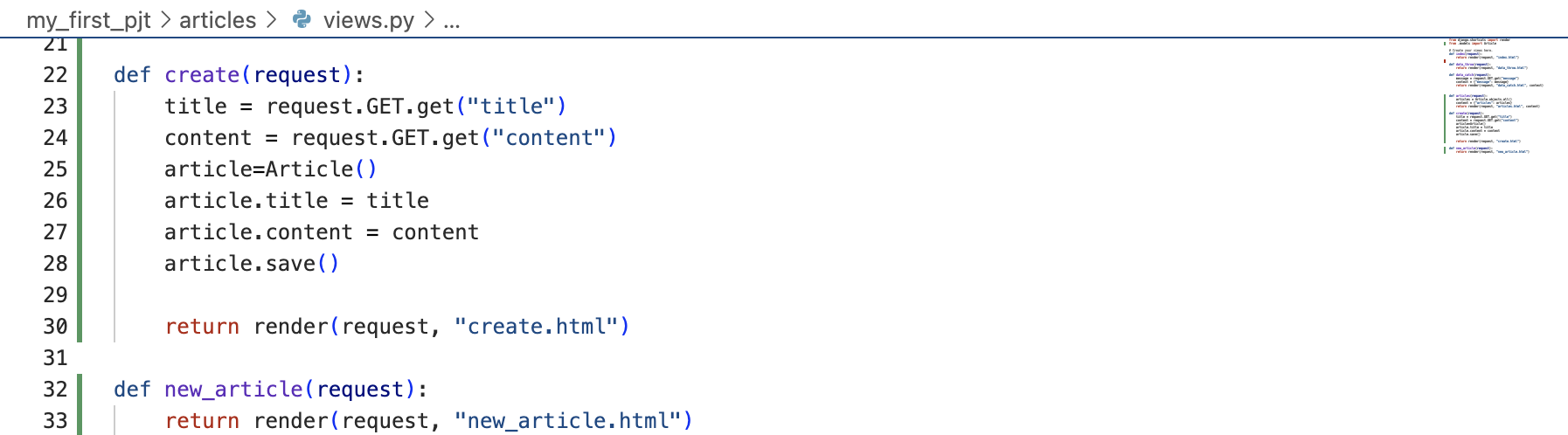
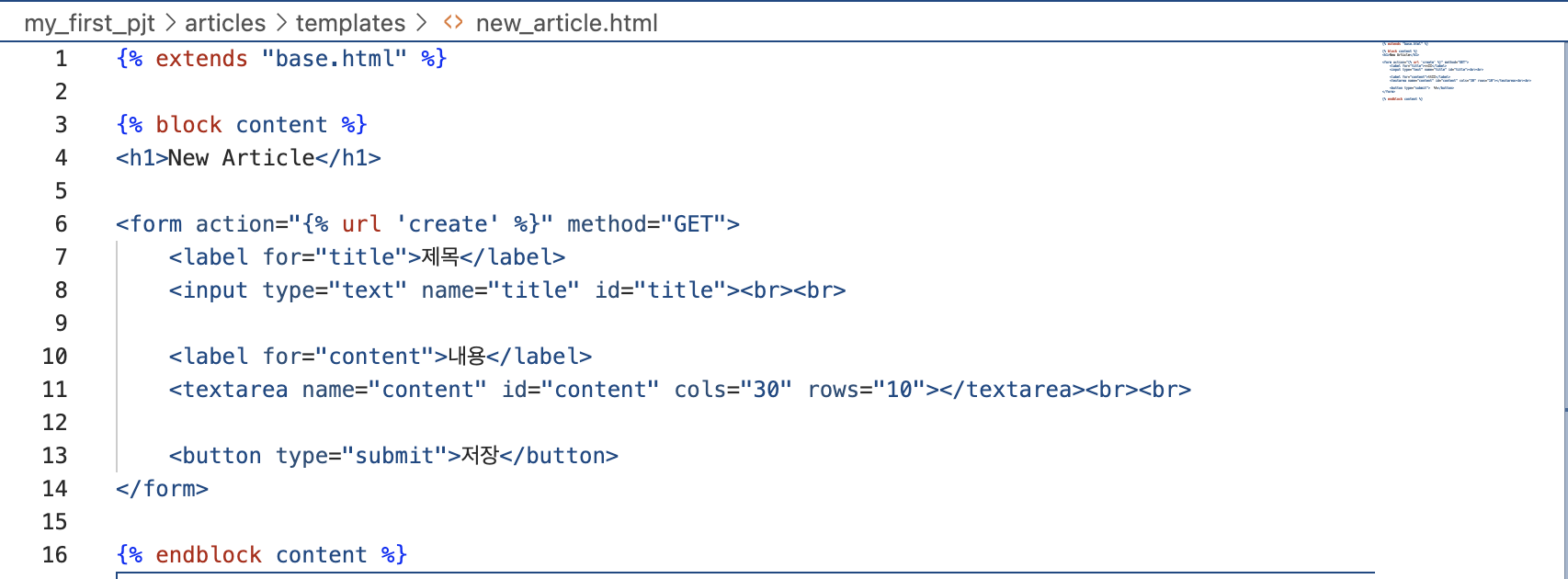
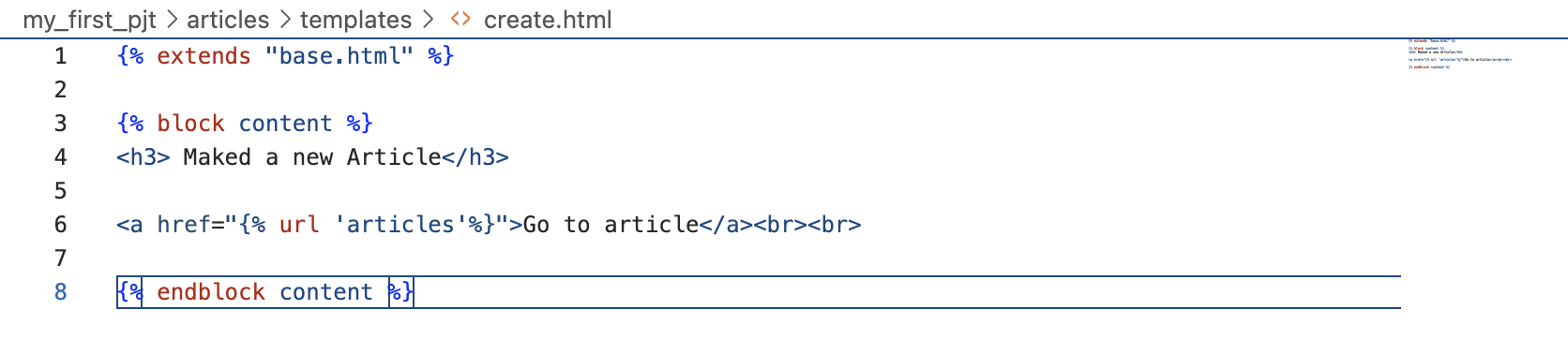
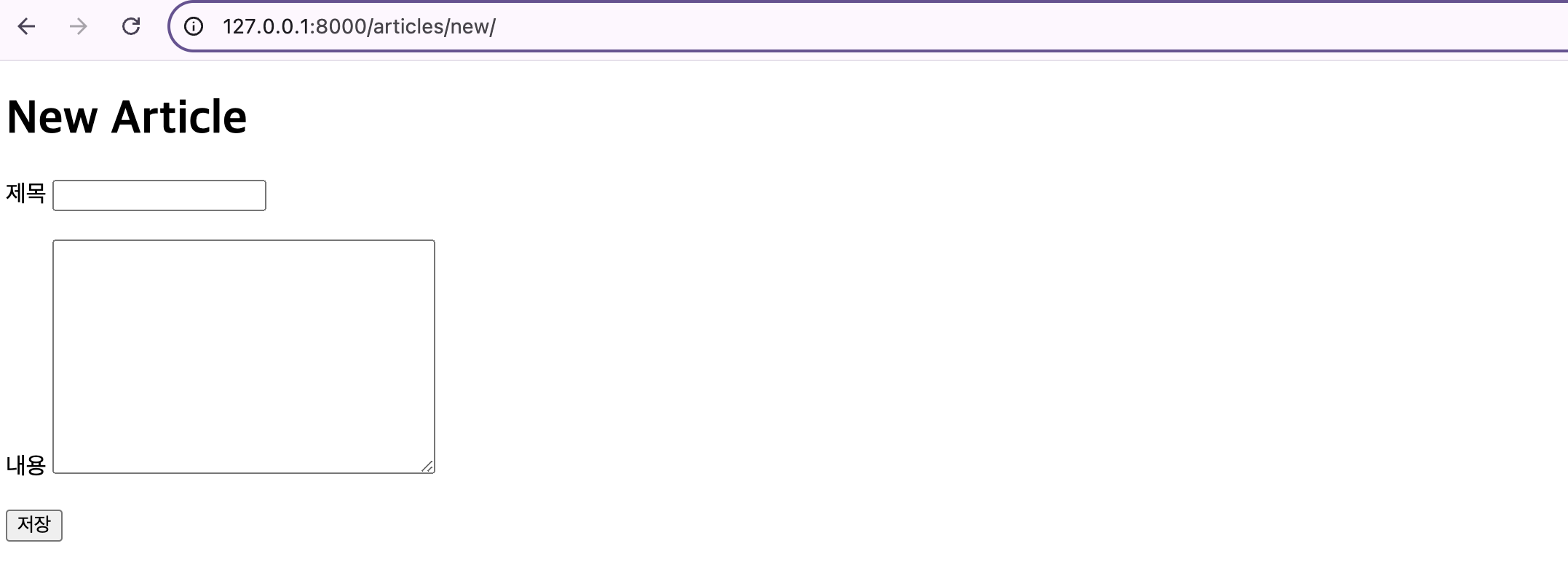
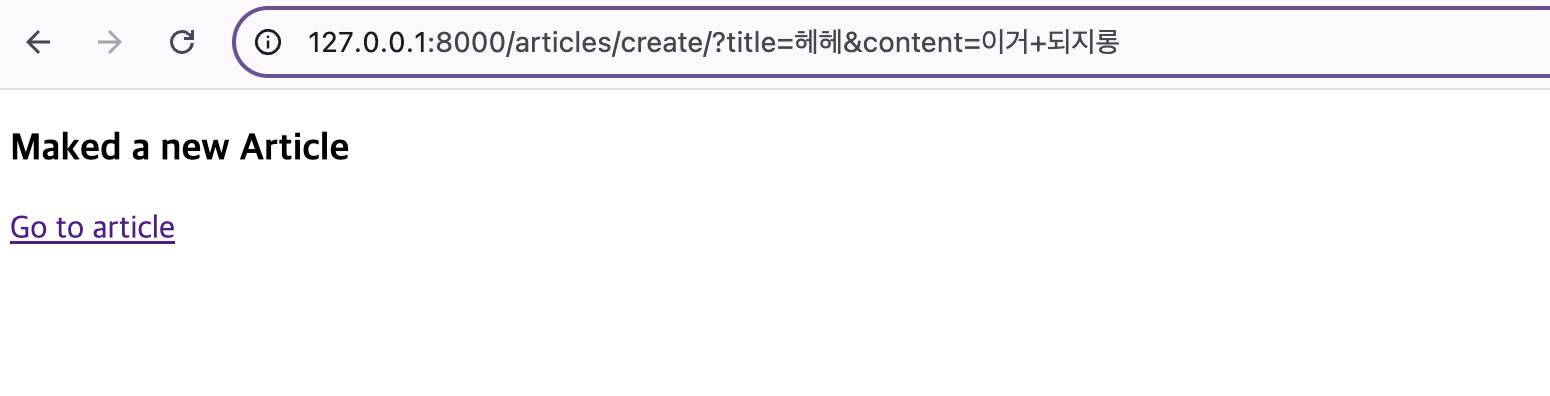
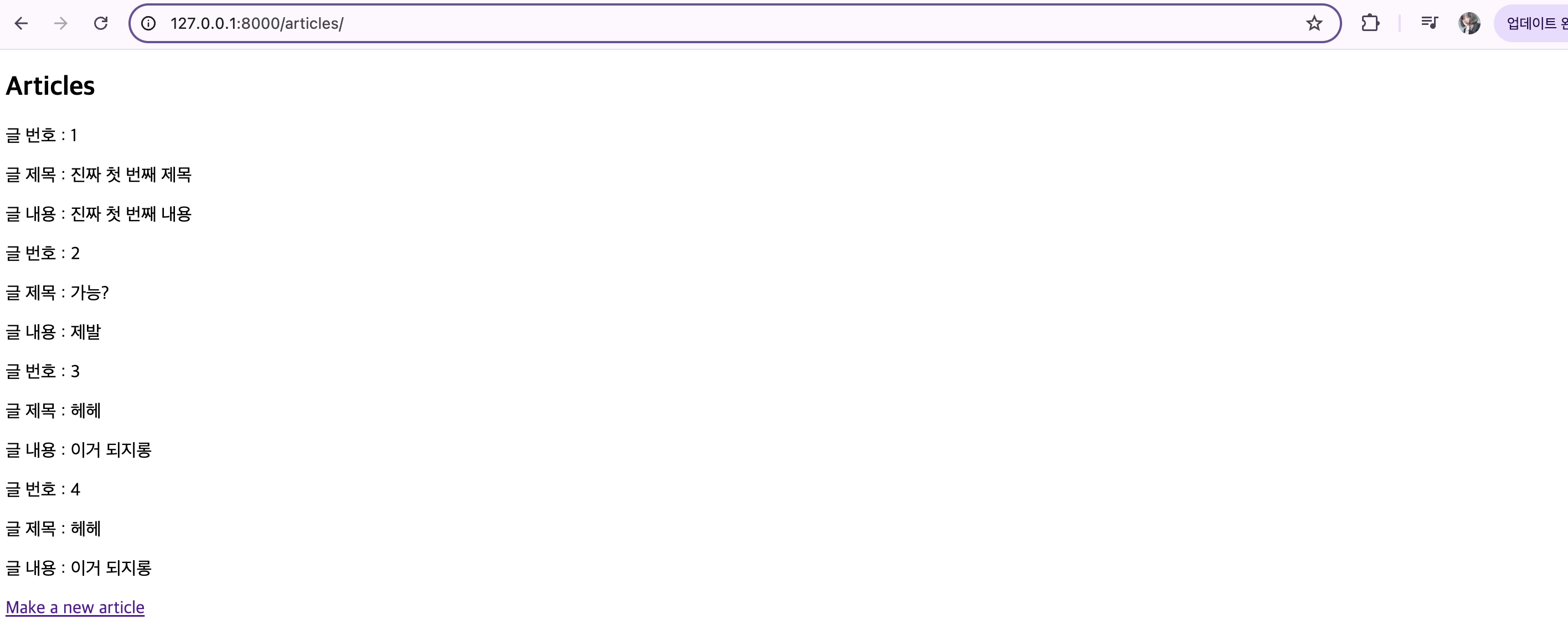
1️⃣ urls.py에 새롭게 추가하기

path('create/', views.create, name="create"),
path('new/', views.new_article, name="new_article"),
- article을 작성할 파일은 new_article
- new_article에서 받은 내용을 보여줄 파일은 create
2️⃣ views.py에 함수 추가하기
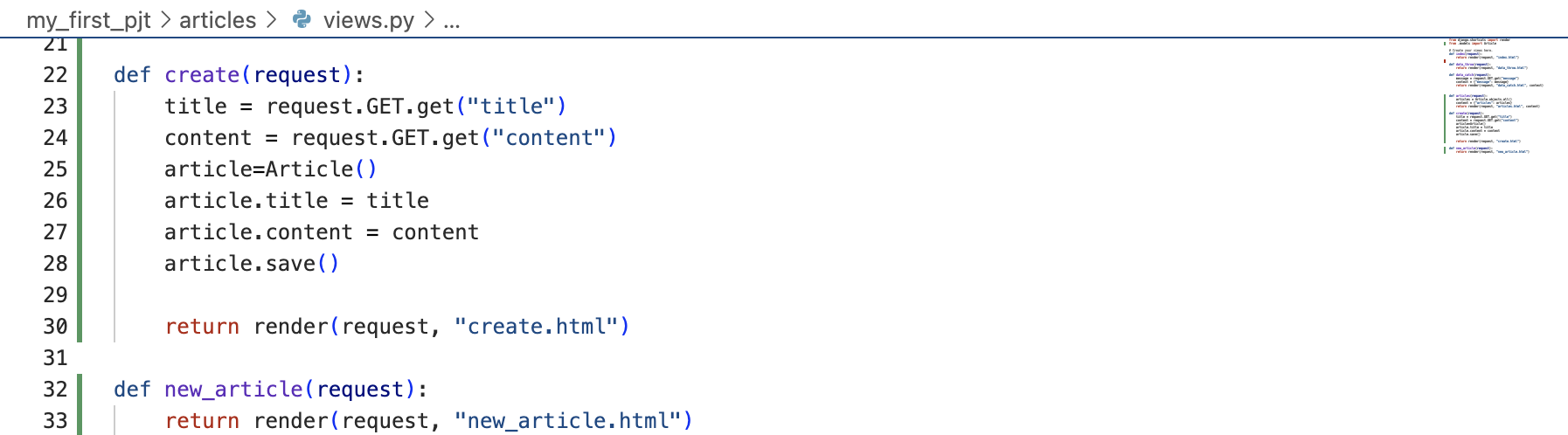
def create(request):
title = request.GET.get("title") # 입력 받은 title을 가져올 곳 설정
content = request.GET.get("content") # 입력 받은 content를 가져올 곳 설정
article=Article() # database 객체 설정
article.title = title # database title 생성
article.content = content # database content 생성
article.save() # database 저장
return render(request, "create.html")
def new_article(request):
return render(request, "new_article.html")
3️⃣ html 작성
new_article.html
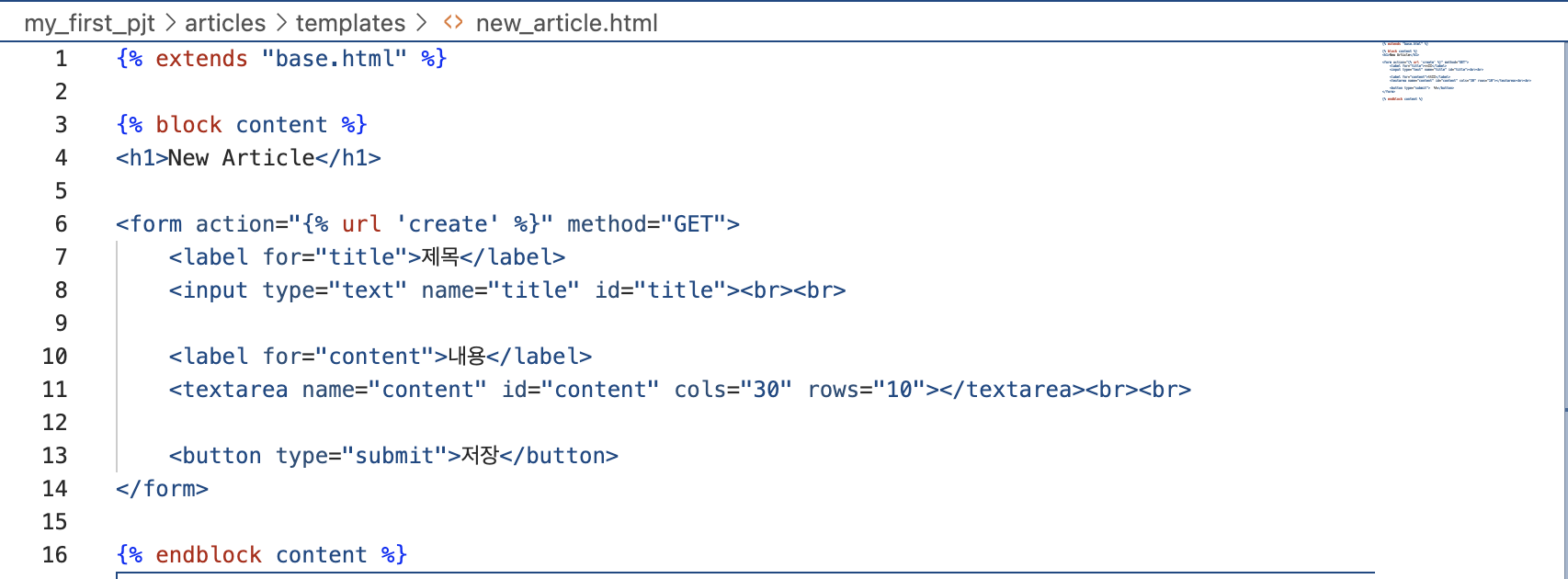
{% extends "base.html" %}
{% block content %}
<h1>New Article</h1>
<form action="{% url 'create' %}" method="GET">
<label for="title">제목</label>
<input type="text" name="title" id="title"><br><br>
<label for="content">내용</label>
<textarea name="content" id="content" cols="30" rows="10"></textarea><br><br>
<button type="submit">저장</button>
</form>
{% endblock content %}
create.html
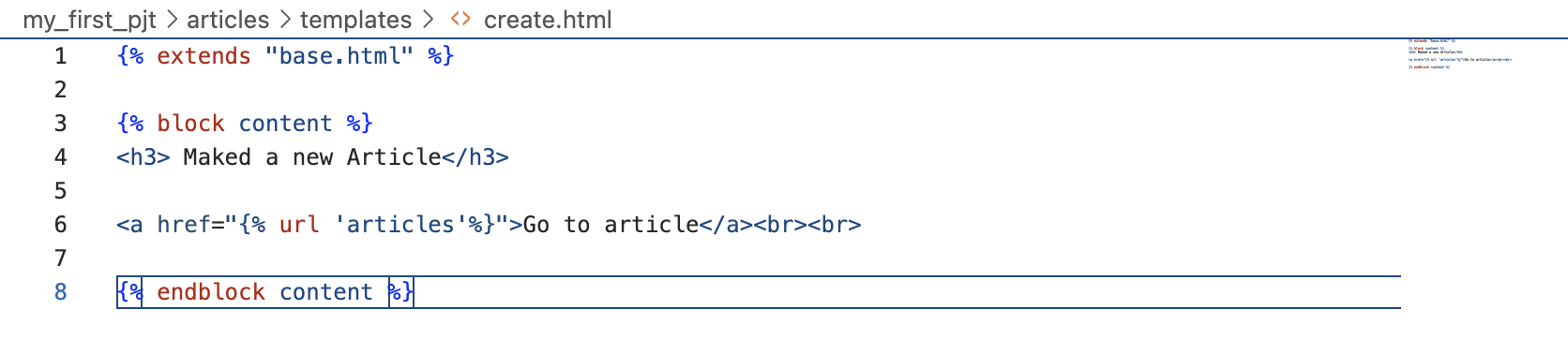
{% extends "base.html" %}
{% block content %}
<h3> Maked a new Article</h3>
<a href="{% url 'articles'%}">Go to Article</a><br><br>
{% endblock content %}
4️⃣ 서버 들어가서 확인하기
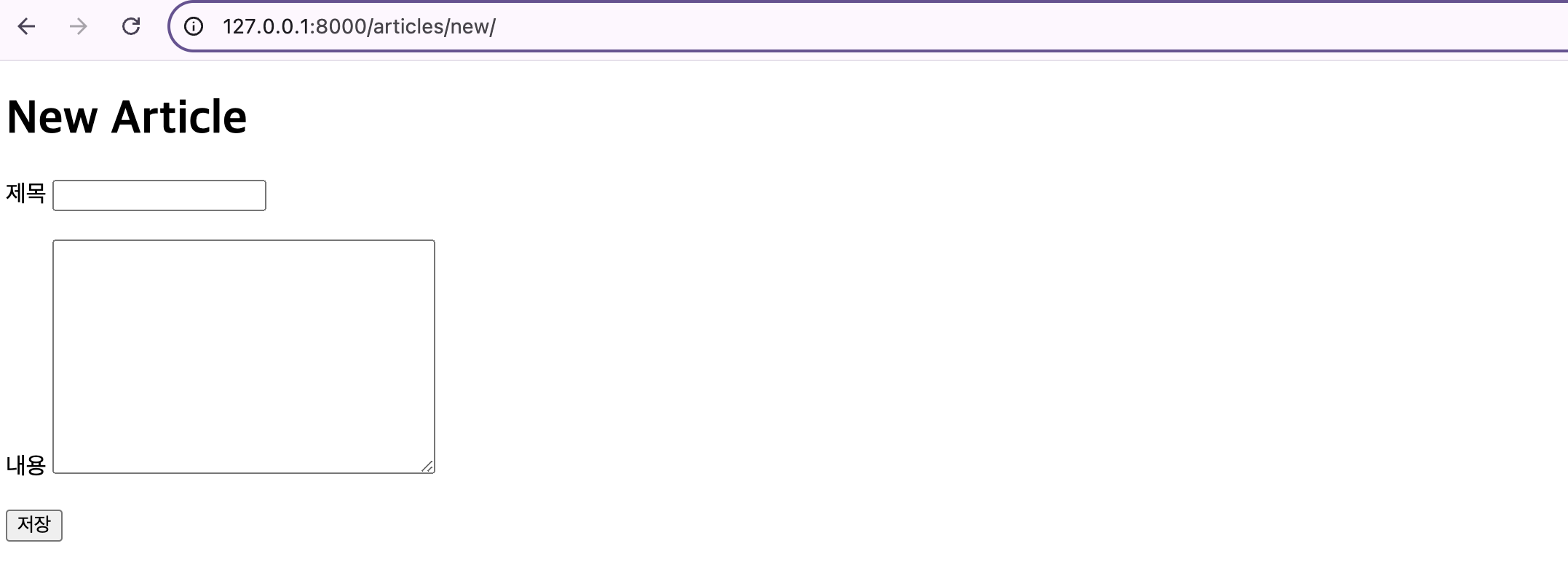
→ new_article.html 정상 작동
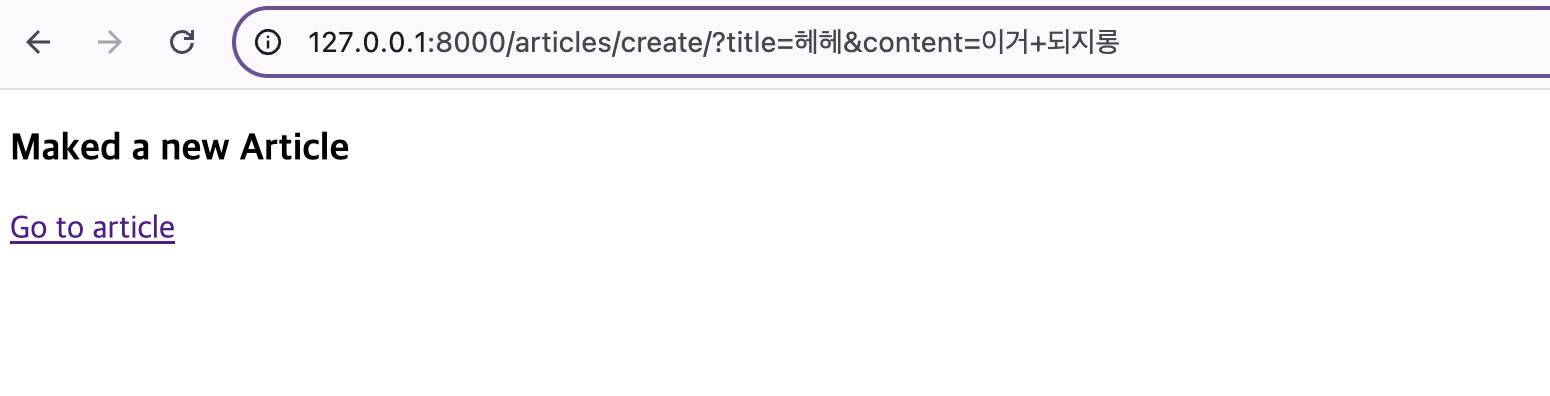
→ create.html 정상 작동
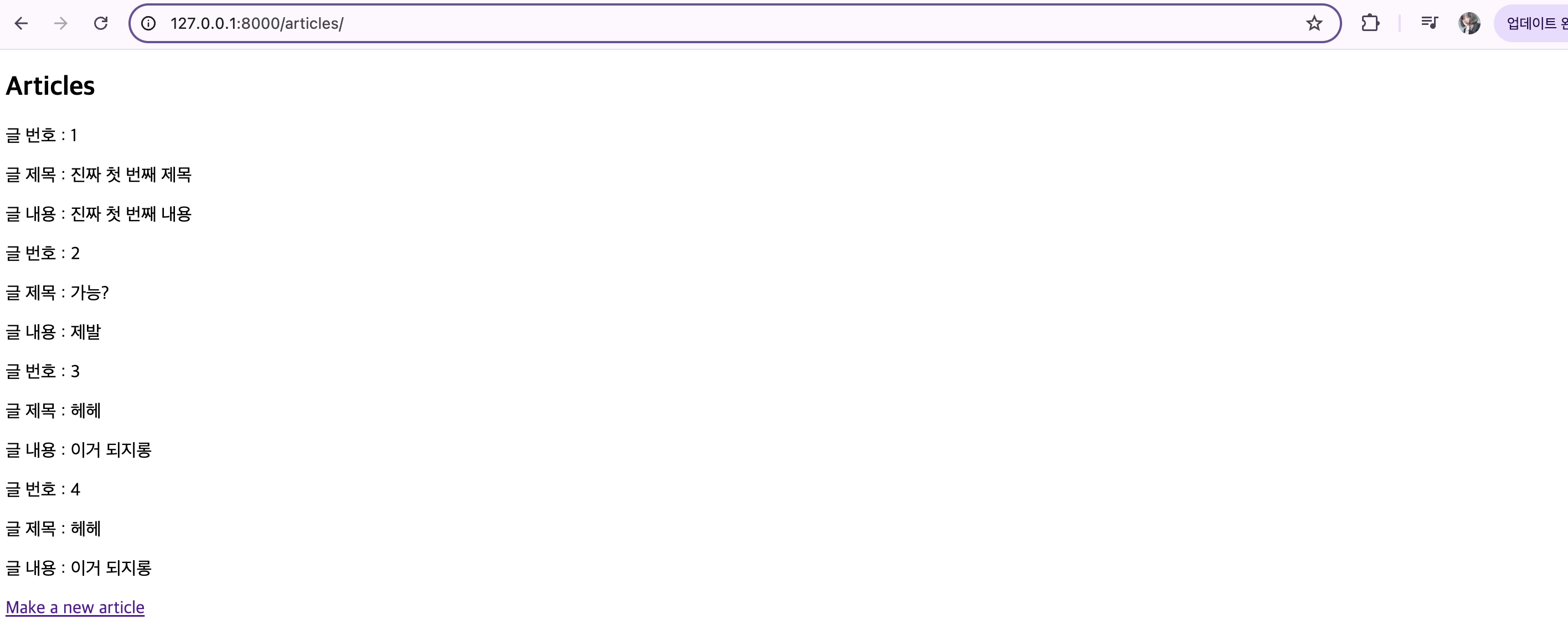
→ article.html 정상 작동
↓ 더 예쁘게 보여주자
더보기
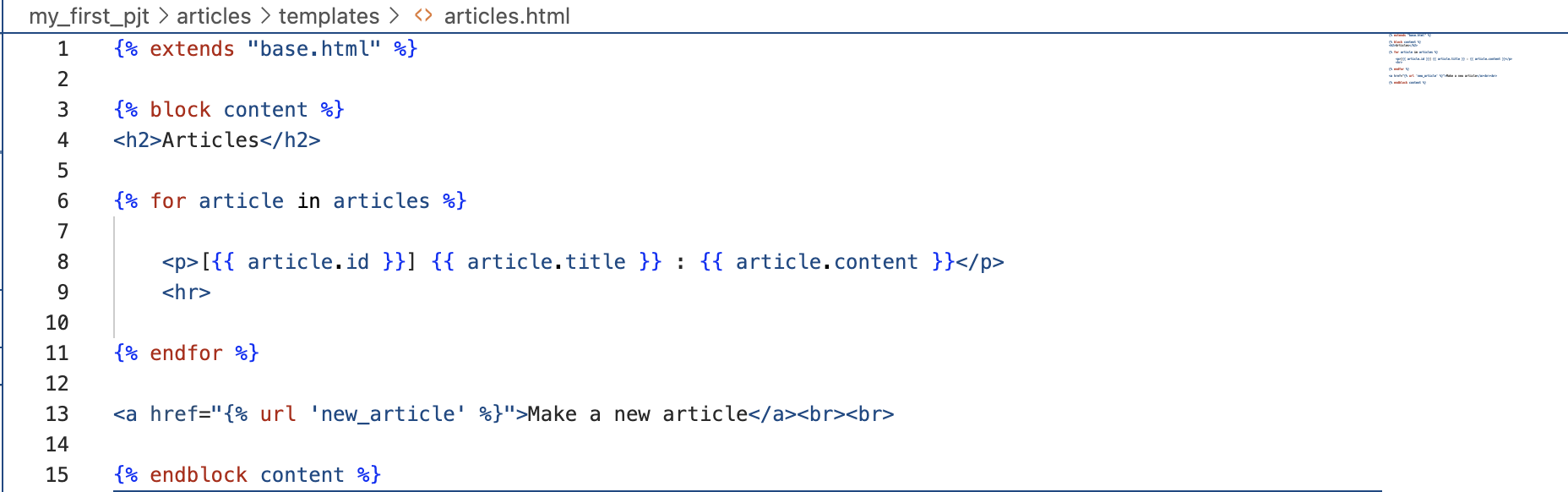

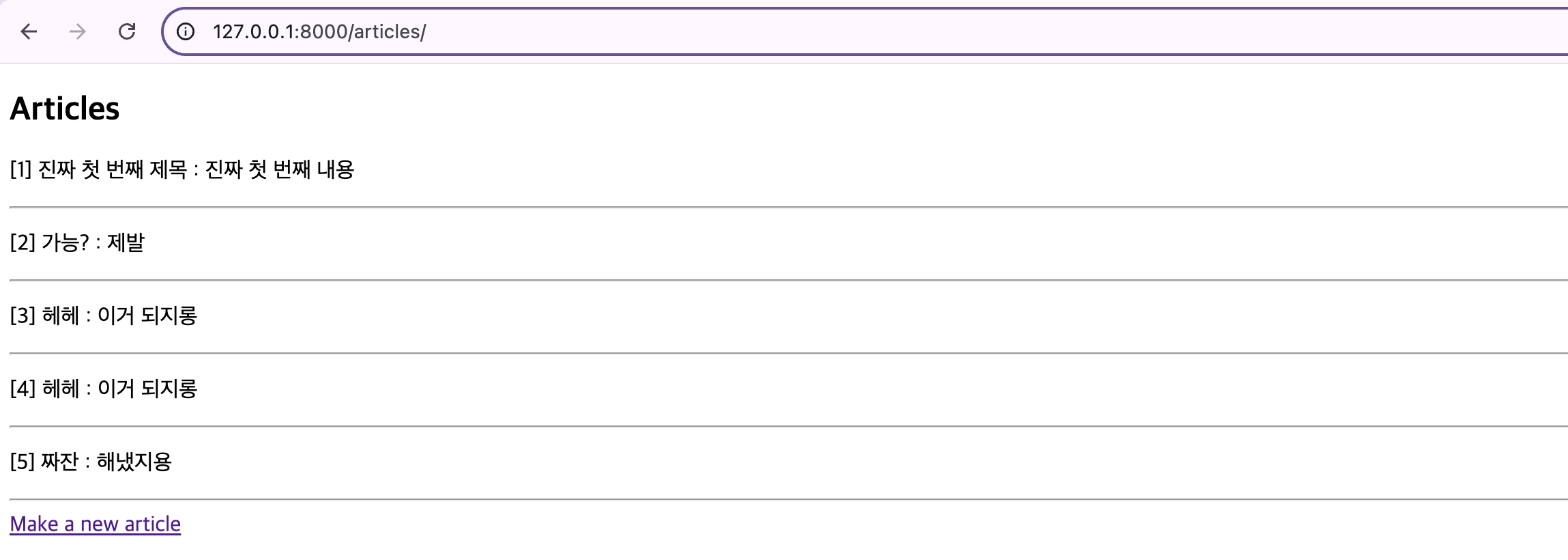
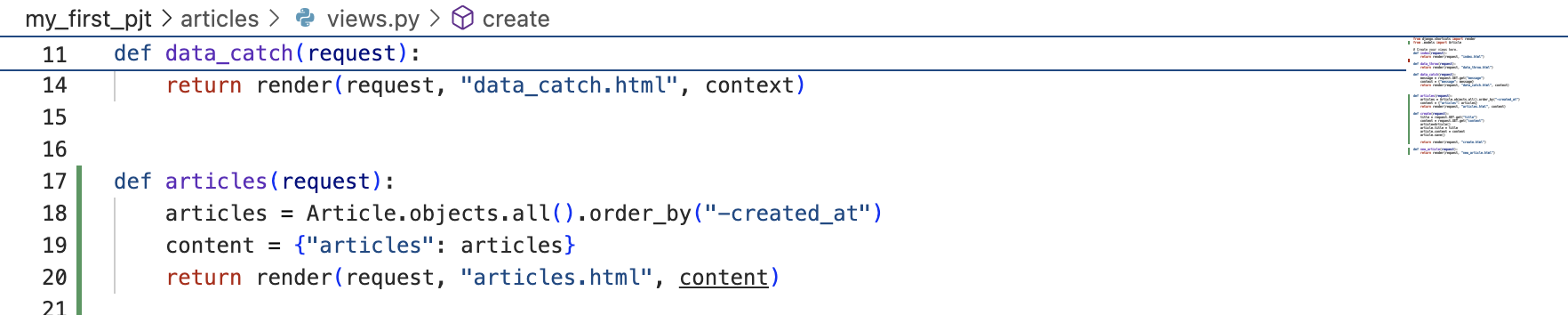
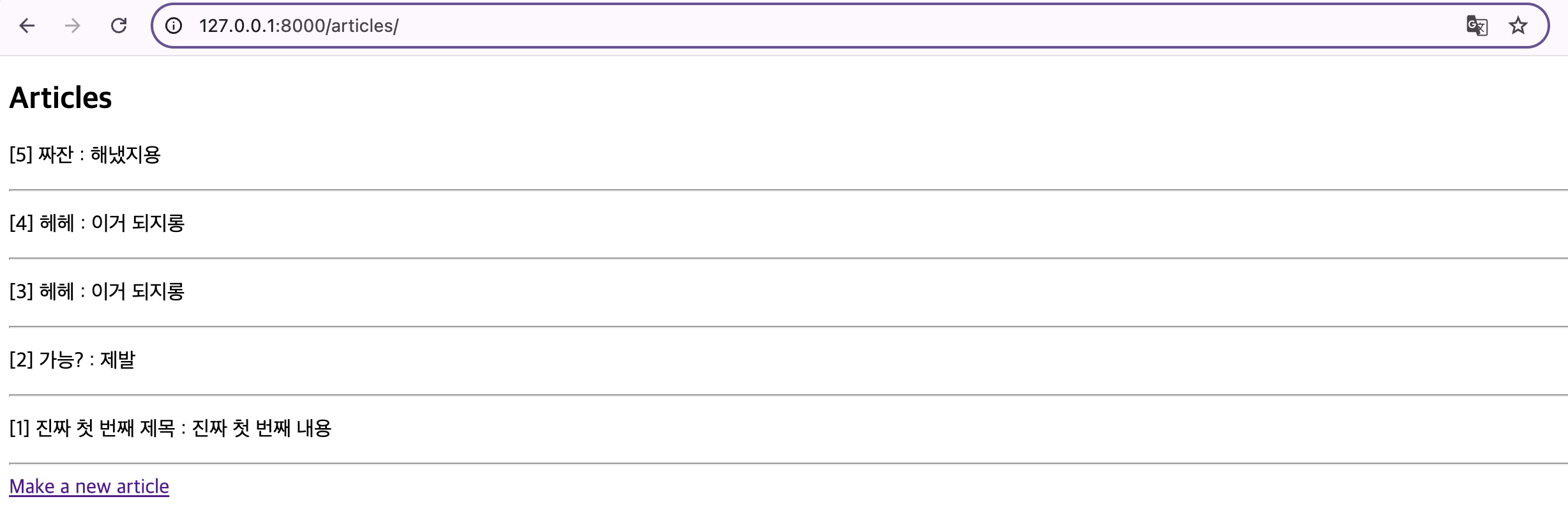
<우선 html 파일을 수정해주었다>
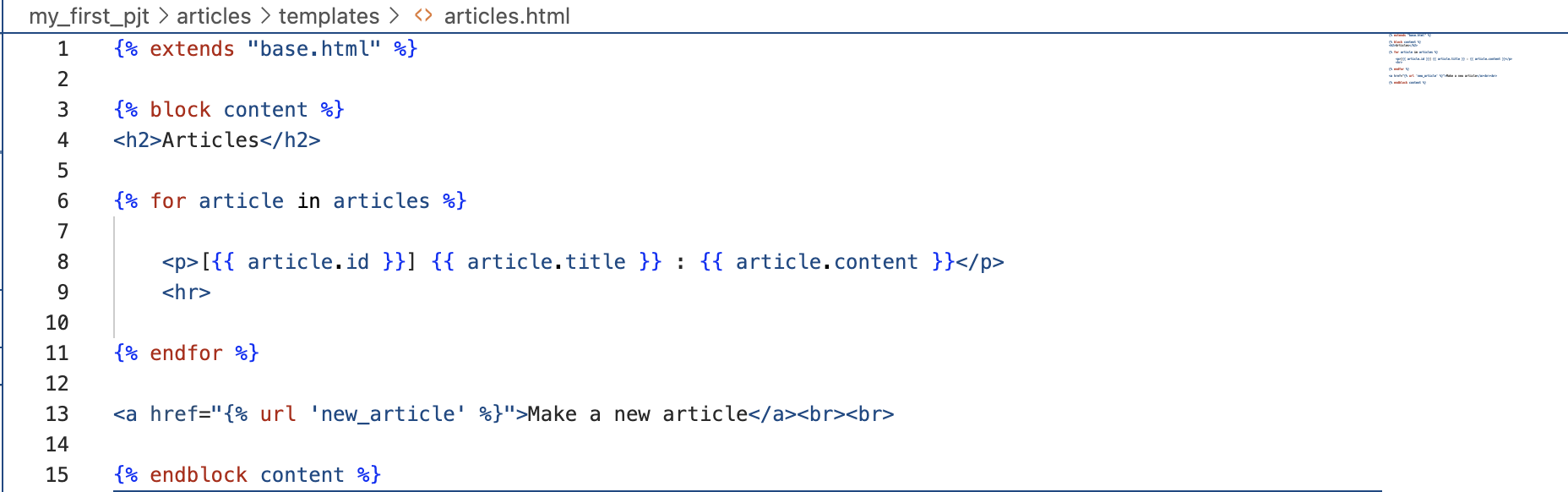
{% extends "base.html" %}
{% block content %}
<h2>Articles</h2>
{% for article in articles %}
<p>[{{ article.id }}] {{ article.title }} : {{ article.content }}</p>
<hr>
{% endfor %}
<a href="{% url 'new_article' %}">Make a new article</a><br><br>
{% endblock content %}
1️⃣ 정렬하기
.order_by("") : 오름차순

def articles(request):
articles = Article.objects.all().order_by("ceated_at")
content = {"articles": articles}
return render(request, "articles.html", content)
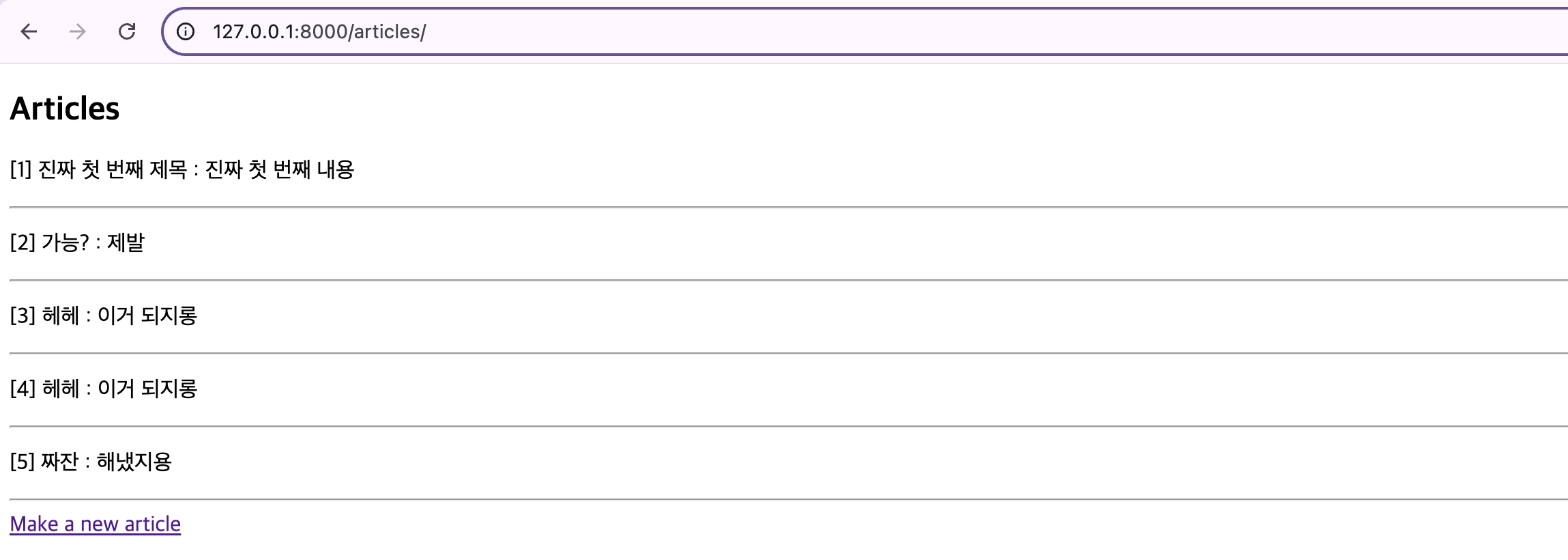
.order_by("-")
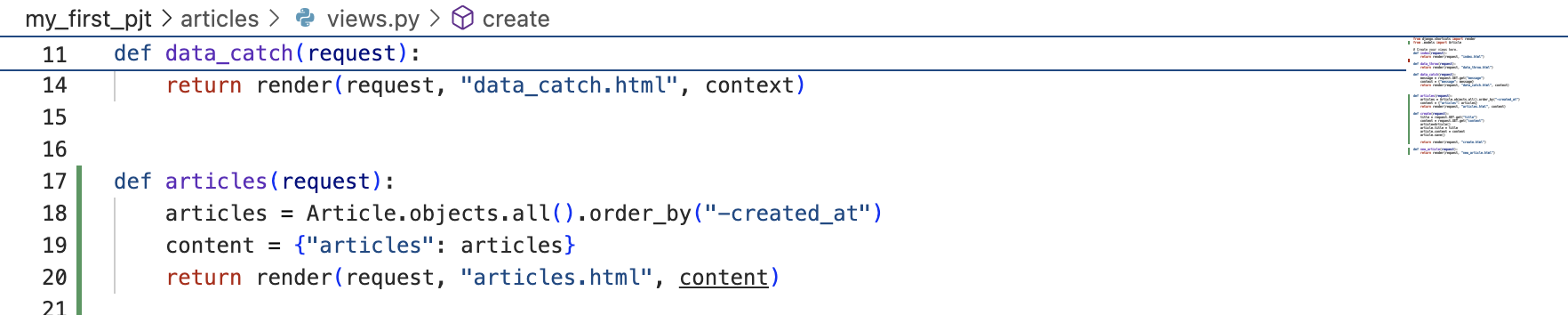
def articles(request):
articles = Article.objects.all().order_by("-created_at")
content = {"articles": articles}
return render(request, "articles.html", content)
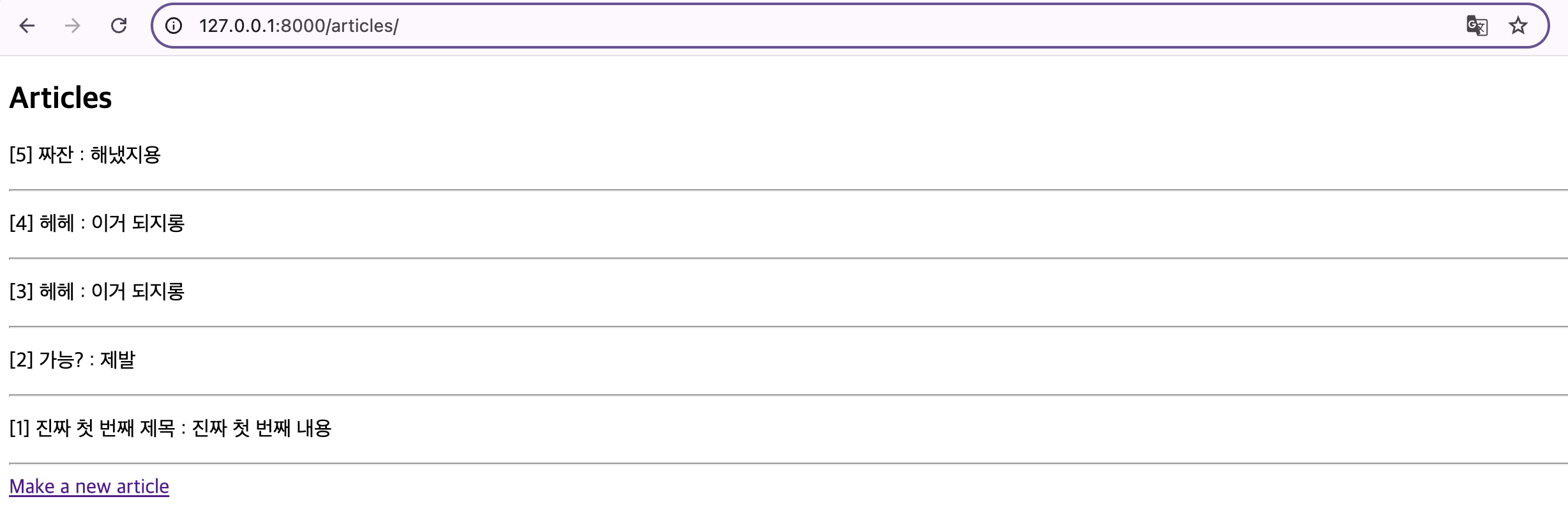
"created_at"=="id"=="pk"
HTTP Method
POST 방식에 대해 알아보자
GET & POST
GET
- 원하는 리소스를 가져오는 데에 사용한다.
- 생성할 때도 사용할 수 있지만(로직상 문제는 없지만), '리소스 조회용으로 사용하자'는 개발 세계에서의 약속이다.
- DB에 변화를 주지 않는 요청임을 의미이다.
- Read에 해당한다.
POST
- 서버로 데이터를 전송할 때 사용한다.
- 특정 리소스를 생성 혹은 수정하기 위해 사용한다.
- DB에 변화를 주는 요청임을 의미한다.
- Create, Update, Delete에 해당한다.
Get Method를 POST Method로 바꿔보자
사이트간 요청 위조 CSRF(Cross-Site-Request-Forgery)
- 유저가 실수로 해커가 작성한 로직을 따라 특정 웹페이지에 수정/삭제등의 요청을 보내게 되는 것
- 좋은 사이트 서버 입장에서는 어디서 온 요청인지 구분이 필요
- 즉, 정말 유저가 의도한 요청인지 검증이 필요
- 아주 나쁜 사이트.com → 요청 → 좋은 사이트.com
- 좋은 사이트 서버 입장에서는 어디서 온 요청인지 구분이 필요
CSRF 해결 방법
{% csrf_token %}
- CSRF Token
- 유저가 서버에 요청을 보낼 때 함께 제공되는 특별한 토큰 값
- 이 토큰은 사용자의 세션과 연결되어 있다.
- 요청이 전송될 때, 함께 제출되며 서버는 요청을 받을 때 이 토큰을 검증하여 요청이 유효한지 확인하는 방식으로 CSRF을 방지한다.
- 쉽게말해 서로 알아볼 수 있는 임의의 비밀번호를 만들어서 주고 받는다는 것
- 일반적으로 GET을 제외한 데이터를 변경하는 Method에 적용한다.
- 유저가 서버에 요청을 보낼 때 함께 제공되는 특별한 토큰 값
↓ {% csrf_token%}으로 해결해보자 == POST Method로 받기
더보기


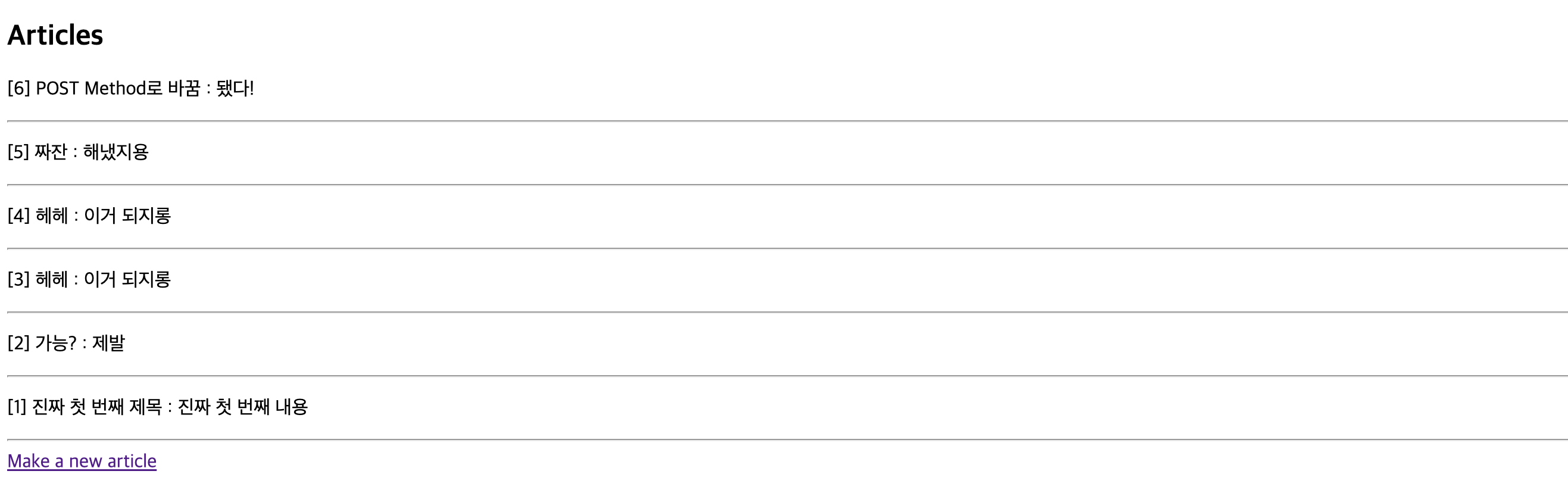
html에서 Method="GET"을 "POST"로 바꾸기

{% extends "base.html" %}
{% block content %}
<h1>New Article</h1>
<form action="{% url 'create' %}" method="POST">
{% csrf_token%}
<label for="title">제목</label>
<input type="text" name="title" id="title"><br><br>
<label for="content">내용</label>
<textarea name="content" id="content" cols="30" rows="10"></textarea><br><br>
<button type="submit">저장</button>
</form>
{% endblock content %}
views.py 수정하기

def create(request):
title = request.POST.get("title")
content = request.POST.get("content")
article = Article(title=title, content=content)
article.save()
서버 실행
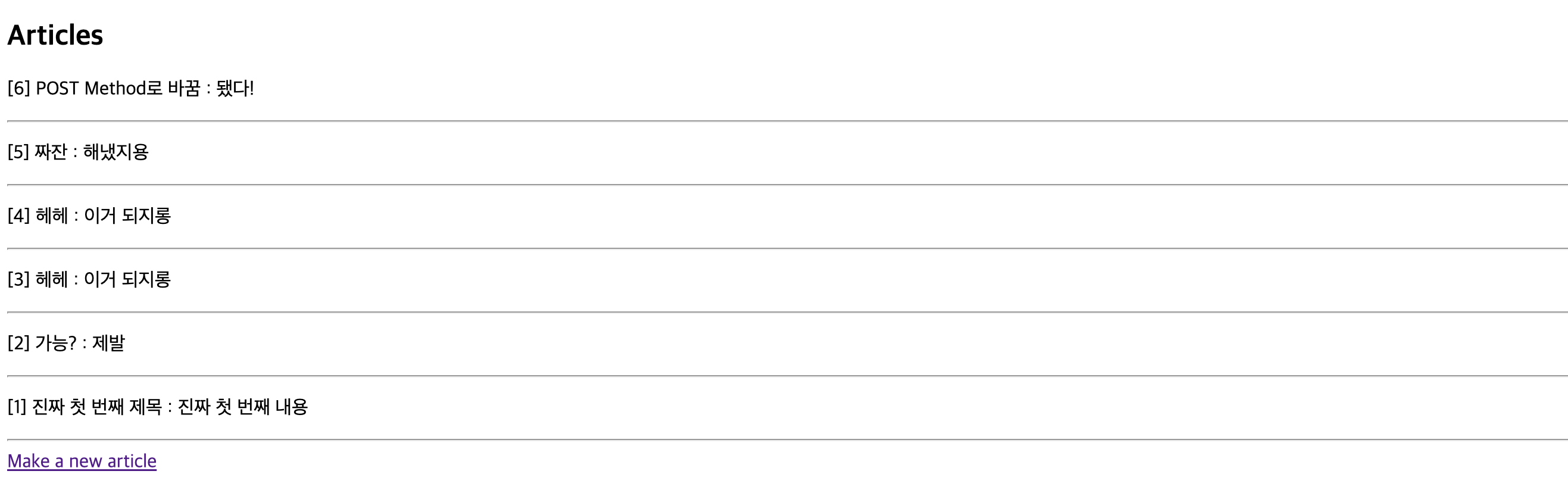
POST 방식 vs GET 방식
HEADER
- HTTP 전송에 필요한 모든 부가정보를 담고있는 부분
- 메시지 크기, 압축, 인증, 요청 클라이언트(웹 브라우저) 정보, 서버 애플리케이션 정보, 캐시 관리 정보 등등 포함
BODY
- 실제 전송할 데이터가 담겨있는 부분으로, 데이터가 없다면 비어있게 된다.
↓ GET 방식 예
더보기
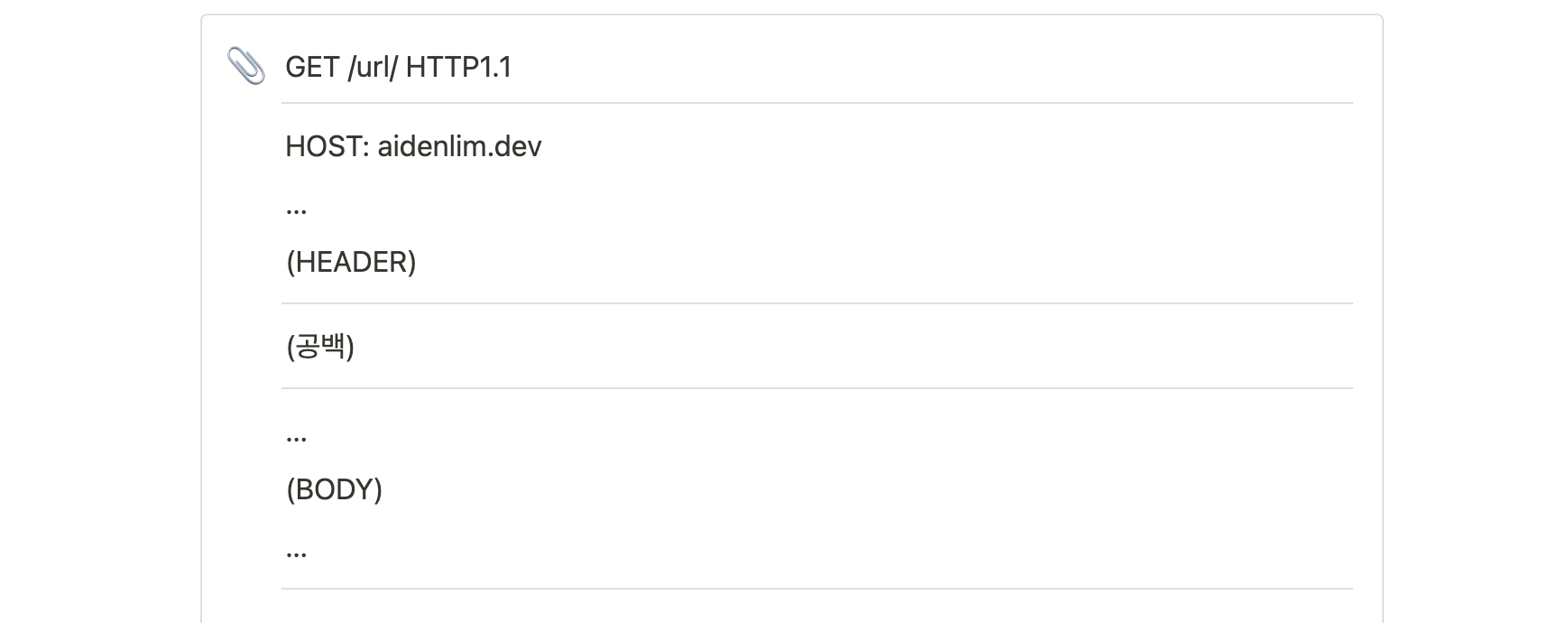
HTTP Request
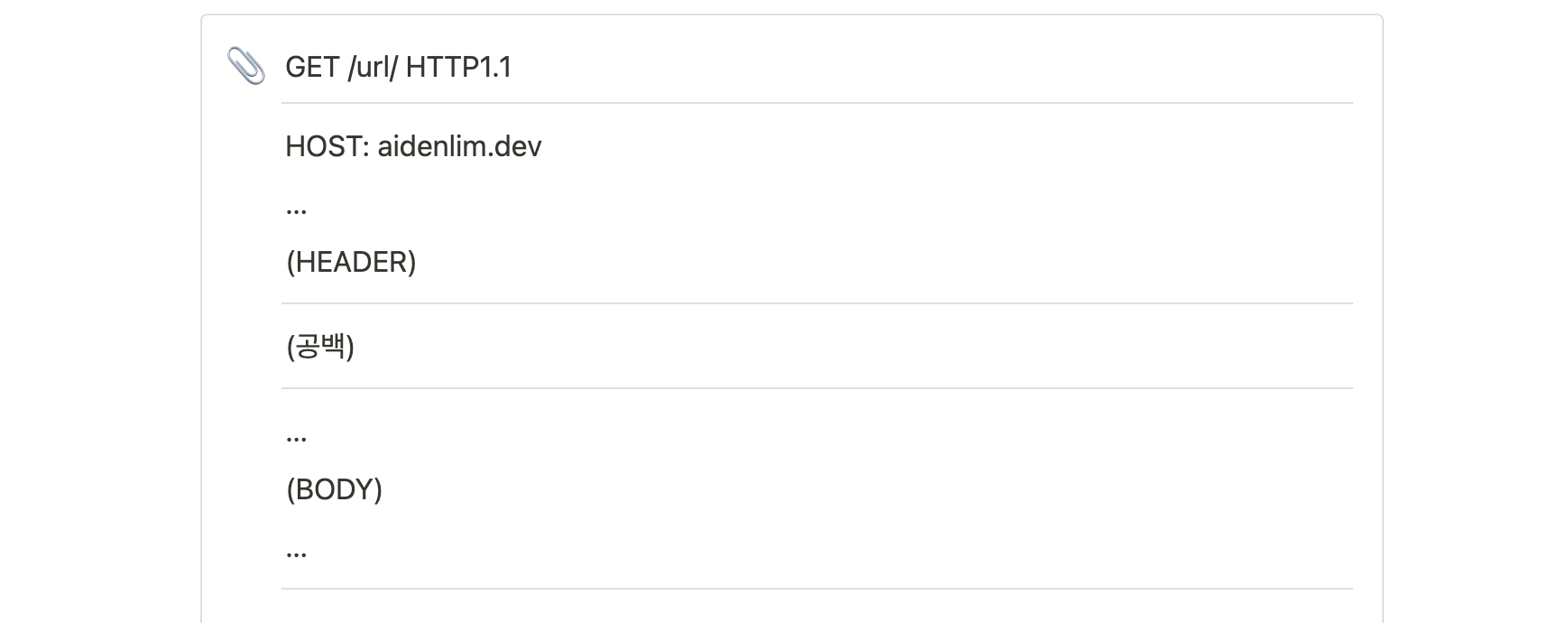
↓ POST 방식 예
더보기
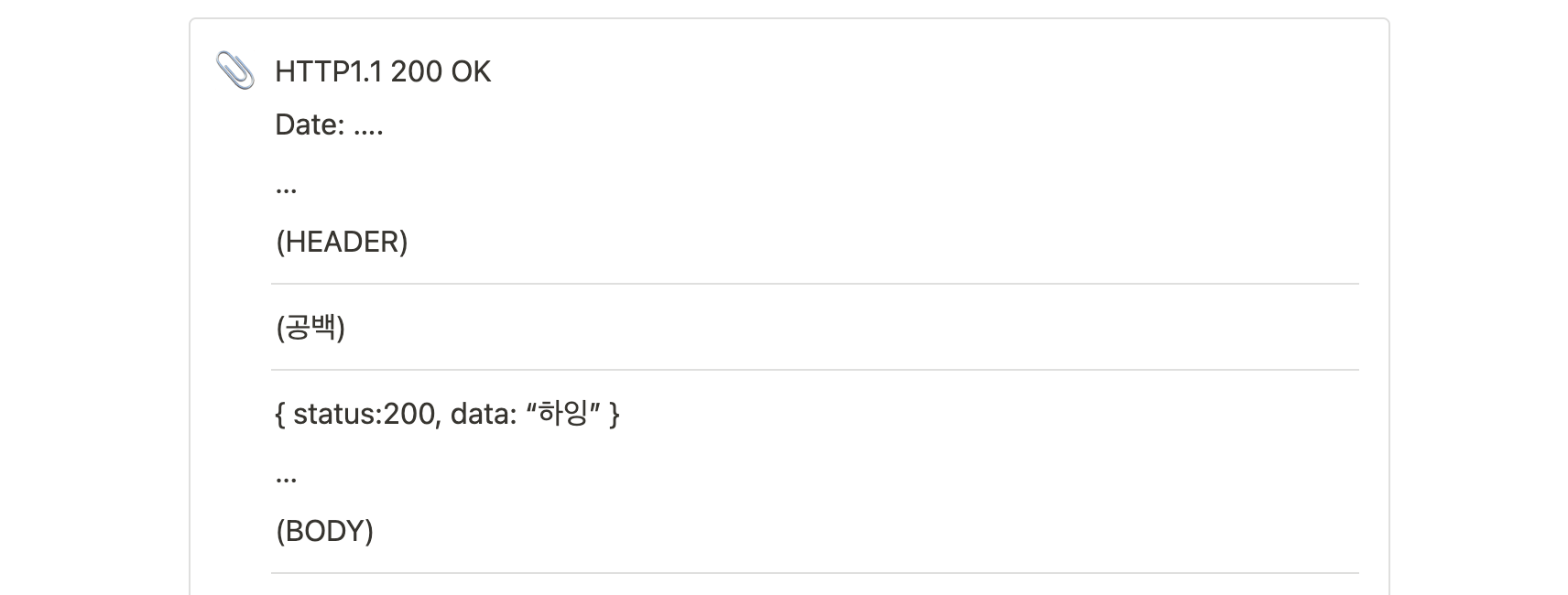
HTTP Response
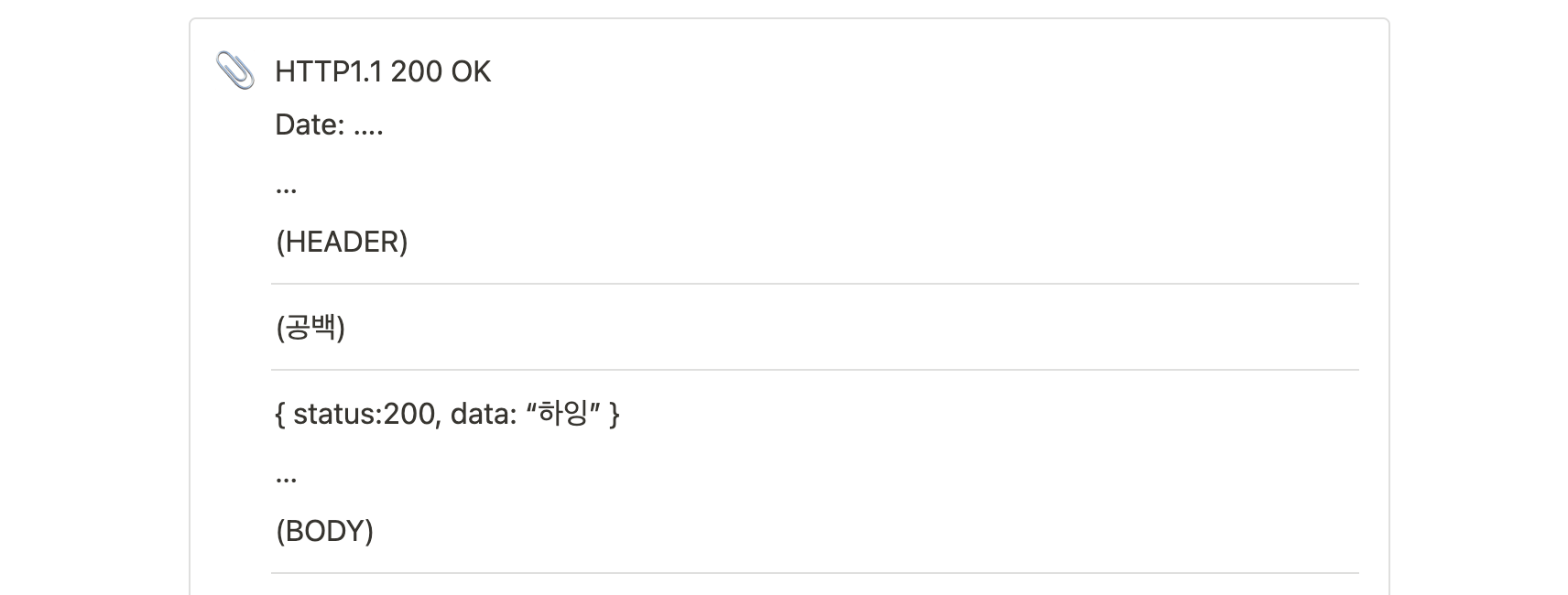
📌 세 줄 요약
- GET은 데이터를 URL에 담아보내고 POST는 BODY에 담아 보낸다.
- 따라서 GET은 데이터 전송에 한계가 있으나, POST는 그렇지 않다.
- POST방식으로 데이터를 전송할 때는 {% csrf_token %}이 필요하다.
'🔥 공부 > 📘 Django 공부' 카테고리의 다른 글
[Django] Django Form Class, Model Form Class (0) | 2025.01.15 |
---|---|
[Django] Django MTV 사용하기 (RUD), 게시판처럼 만들기 (0) | 2025.01.14 |
[Django] Django ORM, CRUD with Shell (0) | 2025.01.14 |
[Django] Django Model, 마이그레이션 (0) | 2025.01.13 |
[Django] 다중 앱과 URL (1) | 2025.01.13 |